[ad_1]
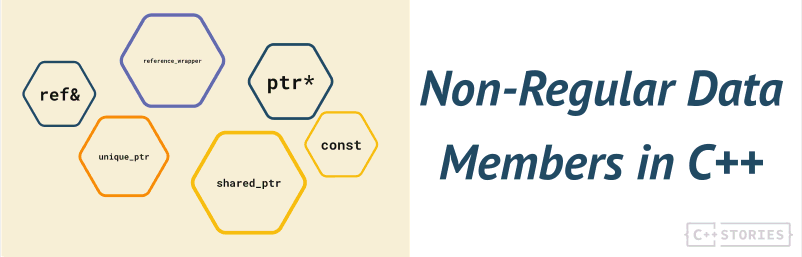
When you’ve got a category with an everyday knowledge member like an integer or string, you’ll get all particular member features out of the field. However how about differing kinds? On this article, we’ll take a look at different classes of members like unique_ptr
, uncooked pointers, references, or const
members.
Introduction
In my guide on “C++ Initialization” I not too long ago wrote a chapter about so-called non-regular knowledge members.
Right here’s a definition based mostly on cppreference – common idea
A sort is common, that’s, it’s copyable, default constructible, and equality comparable. It’s glad by sorts that behave equally to built-in sorts like
int
, and which might be comparable with==
.
For instance:
class Product {
public:
Product() = default;
express Product(std::string identify, unsigned id)
: name_(std::transfer(identify))
, id_(id)
{ }
personal:
std::string name_;
unsigned id_ { };
};
You’ll be able to create, copy, transfer or assign an occasion of the above class. The compiler supplies all particular member features out of the field.
const
When you’ve got a const
knowledge member, then issues get a bit extra difficult:
class ProductConst {
public:
ProductConst() = default;
express ProductConst(const char* identify, unsigned id)
: name_(identify)
, id_(id)
{ }
personal:
std::string name_;
const unsigned id_ { };
};
The situations of the category are:
- default constructible (as
id_
has some default worth), - copy or transfer constructible,
- not assignable. You can not assign a brand new worth. The compiler doesn’t present the copy and transfer task operators.
Pointers
Let’s begin with uncooked pointers… however solely in uncommon instances as they’re problematic and never protected:
class ProductPointer {
public:
ProductPointer() = default;
express ProductPointer(std::string identify, unsigned* pId)
: name_(std::transfer(identify))
, pId_(pId)
{ }
personal:
std::string name_;
unsigned* pId_ { };
};
A uncooked pointer is definitely an everyday object, so you may copy or change it. However the semantics of the category with that member sort is a little more complicated:
- An occasion of
ProductPointer
is default constructible, ProductPointer
has transfer operations- However the copy is problematic as it will likely be solely a shallow copy – you may create many situations pointing to the identical useful resource (like an allotted reminiscence block). Nonetheless, it will likely be a difficulty to delete it and notify different house owners safely.
It’s finest to depend on good pointers, relying on this system’s necessities.
class ProductUniquePointer {
public:
ProductUniquePointer() = default;
express ProductUniquePointer(std::string identify, unsigned Id)
: name_(std::transfer(identify))
, pId_(std::make_unique<unsigned>(Id)) // make a replica
{ }
personal:
std::string name_;
std::unique_ptr<unsigned> pId_;
};
An occasion of ProductUniquePointer
has:
- default constructor
- default transfer operations
- deleted copy constructor and replica task
And the shared_ptr
model:
class ProductSharedPointer {
public:
ProductSharedPointer() = default;
express ProductSharedPointer(std::string identify, unsigned Id)
: name_(identify)
, pId_(std::make_shared<unsigned>(Id)) // make a replica
{ }
personal:
std::string name_;
std::shared_ptr<unsigned> pId_;
};
This time an occasion of ProductSharedPointer
has:
- default constructor
- default transfer operations
- default copy operations, however they’re shallow. Nonetheless, “shallow” could be high-quality for the shared pointer, because the useful resource will likely be safely shared throughout many homeowners.
In each instances, I create a brand new pointer and replica the id
argument.
Learn extra about good pointers in:
References
It’s a sophisticated factor:
class ProductRef {
public:
express ProductRef(std::string identify, unsigned& id)
: name_(std::transfer(identify))
, idRef_(id)
{ }
personal:
std::string name_;
unsigned& idRef_;
};
Cases of the category have:
- no default constructor obtainable, a reference can’t be null/empty
- the compiler supplies a default copy and transfer constructors
- task operator is deleted, as you can not rebind a reference
Alternatively, you may strive utilizing std::reference_wrapper
, which behaves like a reference, however could be rebounded to a unique object.
Remaining desk
Because of sort traits from the Customary Library, we are able to have a fast check displaying the properties of such courses. The core perform is:
template <typename T>
void ShowProps() ";
cout << "transfer constructible " << is_move_constructible_v<T> << 'n';
Utilizing the above perform template, I generated the next desk:
Non-static knowledge member sort | Default ctor | Copy ctor | Copy assign | Transfer ctor | Transfer assign |
---|---|---|---|---|---|
copyable, assignable, “common” | sure | default | default | default | default |
const knowledge member |
no, except default worth is ready | default | customized solely | default | customized solely |
pointer sort | sure | default(shallow!) | default(shallow!) | default | default |
std::unique_ptr |
sure | customized solely | customized solely | default | default |
std::shared_ptr |
sure | default, shallow, however could be protected | default, additionally shallow | default | default |
reference sort | no | default(shallow) | customized solely | default | customized solely |
std::reference_wrapper |
no | default (shallow!) | default(shallow) | default | default |
For instance, when your class has a const
knowledge member, the default constructor is unavailable (except you assign some default worth), the compiler can present the copy and the transfer constructors, however default task operators are unavailable. “Customized solely” implies that the compiler can not generate a default implementation, and the person has to offer some customized implementation.
Run the instance @Compiler Explorer
Abstract
On this textual content, we coated a couple of forms of (non-static) knowledge members which may trigger points within the implementation. Some block a default constructor, and a few copy or task operations. I hope that this text gave you some helpful overview and fundamental concepts on the best way to work with situations of such courses. If you wish to know extra, then take a look at my guide that incorporates much more examples and discussions: C++ Initialization Story by Bartłomiej Filipek (new free replace later this week!).
Again to you
- Do you employ references, uncooked pointers non-moveable objects as knowledge members?
- Do you may have strategies to keep away from them?
- What different “particular” classes of objects do you employ in your courses?
Share your suggestions within the feedback beneath.
[ad_2]