What is Memory Management in C Language?
What is Array in C Language?
What is Control Statements in C Language?
What is File Handling in C Language?
What is Pointer in C Language?
What is Function in C Language?
What is String in C Language?
What is Variable Declaration in C?
What is Storage Classes in C Language?
What is the Scope of Variables in C Language?
What is Preprocessor in C Language?
What is Operators in C Language?
What is Data Types in C Language?
What is Input and Output Functions in C Language?
Introduction to C Language
Why c is called procedure-oriented programming language?
How to Learn the C and C++ Languages: The Ultimate List
After All These Years, the World is Still Powered by C Programming
History of C programming and its uses
Is the War Between C and C++ Over?
Here is a Hello World program in C
Install C on Windows
What is C programming?
C Data Types
Difference between C and C++
What is Memory Management in C Language?
Fahad Ali
•
March 1, 2021
•
C Language
•
No Comments
The C language provides many functions contained in header files to handle memory allocation and management. This tutorial briefly describes how to manage your program memory using various functions and their respective header files.
All programming languages deal with memory in the system. All variables require the specified amount of memory. The program itself needs memory to store its own program, temporary memory to store intermediate values, etc. Therefore, great care must be taken when managing memory. The memory location assigned to a program or variable must not be used by another program or variable. He.nce C provides two ways to allocate memory for variables and programs. They are static and dynamic memory allocation. With static memory allocation, memory is allocated at compile time and is the same throughout the program. There are no changes to the amount of memory or the memory location. However, with dynamic memory allocation, memory is allocated at run time and you can increase or decrease the amount of memory allocated or completely free memory when it is not in use. You can reallocate memory as needed. Therefore, dynamic memory allocation gives you the flexibility to use memory efficiently.
Static Memory Allocation:
With the static memory allocation technique, memory allocation is done at compile time and remains the same during program execution. There are no changes to the amount of memory or the memory location.
Dynamic Memory Allocation:
With dynamic memory allocation technology, memory allocation is done at program execution time, with the ability to increase or decrease the amount of memory allocated, and to free or free memory when it is not needed or used . You can also reallocate memory if you want. So it is more advantageous and can manage memory efficiently.
There are several functions that allocate memory to a variable at run time and vary the memory size of the variable. The best examples of dynamic memory allocation are pointers, structures, and arrays. I don’t know the number and type of variables used here. You can use the following functions to allocate memory at run time and determine the variable type.
The <stdlib.h> library has functions responsible for dynamic memory management.
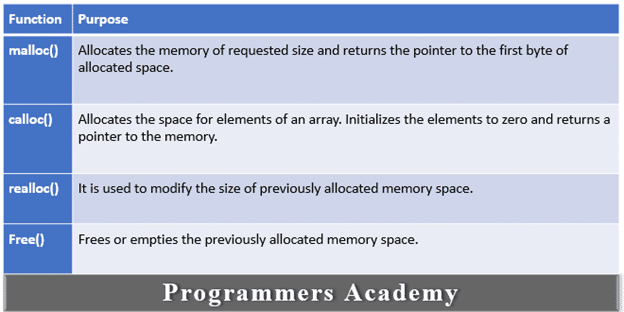
Let’s talk about the above function and its application.
malloc () function:
The C malloc () function represents the memory allocation. This is a function that is used to dynamically allocate blocks of memory. Allocates a memory space of the specified size and returns a null pointer to the memory location. This means that you can assign the C malloc () function to any pointer. The Malloc () function can be used not only for character data types, but also for complex data types, such as structures.
Syntax of malloc() Function:
ptr = (cast_type *) malloc (byte_size);
Where,
ptr is a pointer of cast_type.
The malloc() function returns a pointer to the allocated memory of byte_size.
For example:
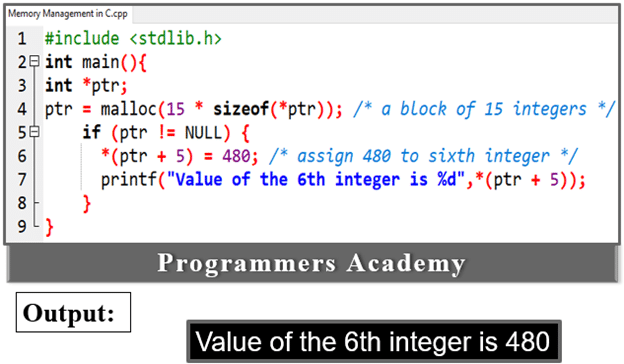
Here Code:
#include <stdlib.h>
int main(){
int *ptr;
ptr = malloc(15 * sizeof(*ptr)); /* a block of 15 integers */
if (ptr != NULL) {
*(ptr + 5) = 480; /* assign 480 to sixth integer */
printf(“Value of the 6th integer is %d”,*(ptr + 5));
}
}
free() Function:
Variable memory is deallocated automatically at compile time. Dynamic memory allocation requires that you explicitly deallocate memory. Otherwise, you may get an out of memory error.
The free () function is called to free / unallocate memory in C. By freeing up the program memory, you can use it later.
For example:
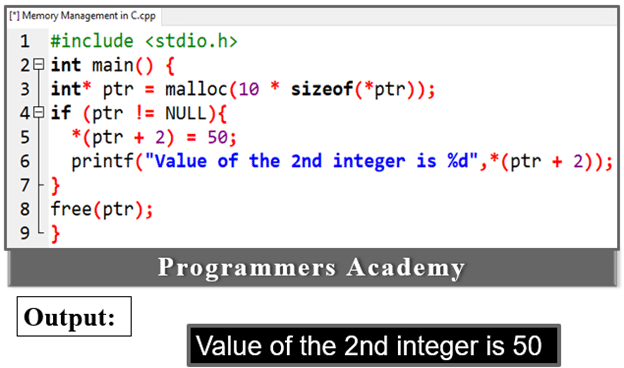
Here Code:
#include <stdio.h>
int main() {
int* ptr = malloc(10 * sizeof(*ptr));
if (ptr != NULL){
*(ptr + 2) = 50;
printf("Value of the 2nd integer is %d",*(ptr + 2));
}
free(ptr);
}
calloc() Function:
The calloc () function represents continuous assignment. This is a dynamic memory allocation function used to allocate memory for complex data structures such as arrays and structures.
Syntax of calloc() Function:
ptr = (cast_type *) calloc (n, size);
For example:
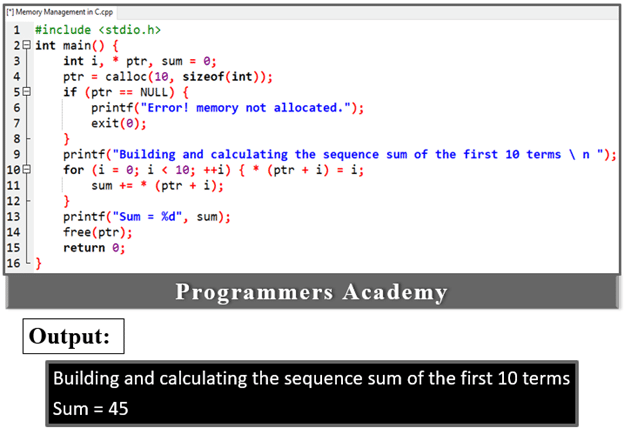
Here Code:
#include <stdio.h>
int main() {
int i, * ptr, sum = 0;
ptr = calloc(10, sizeof(int));
if (ptr == NULL) {
printf("Error! memory not allocated.");
exit(0);
}
printf("Building and calculating the sequence sum of the first 10 terms n ");
for (i = 0; i < 10; ++i) { * (ptr + i) = i;
sum += * (ptr + i);
}
printf("Sum = %d", sum);
free(ptr);
return 0;
}
realloc() Function:
You can use the realloc () function to add memory size to already allocated memory. Expands the current block, leaving the original content intact. C’s Realloc () represents memory reallocation.
You can also use realloc () to reduce the size of pre-allocated memory.
Syntax of realloc() Function:
ptr = realloc (ptr,newsize);
For example:
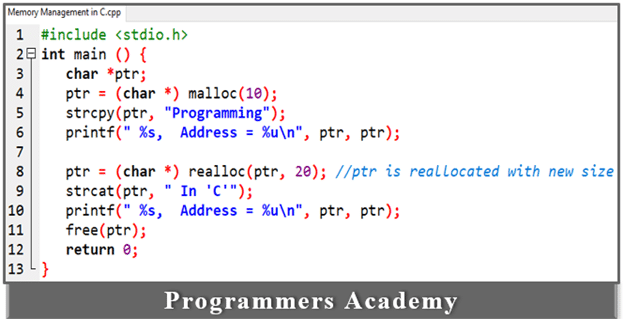
Here Code:
#include <stdio.h>
int main () {
char *ptr;
ptr = (char *) malloc(10);
strcpy(ptr, "Programming");
printf(" %s, Address = %un", ptr, ptr);
ptr = (char *) realloc(ptr, 20); //ptr is reallocated with new size
strcat(ptr, " In 'C'");
printf(" %s, Address = %un", ptr, ptr);
free(ptr);
return 0;
}
Conclusion:
You can manage memory dynamically by creating memory blocks in the heap as needed. With dynamic C memory allocation, memory is allocated at run time. Dynamic memory allocation allows you to manipulate strings and arrays that are flexible in size and can be changed programmatically at any time. Required if you don’t know how much memory a particular structure will take up. Malloc () from C is a dynamic memory allocation function that represents a memory allocation that locks a particular size of memory initialized to a garbage value. Calloc () in C is a contiguous memory allocation function that allocates multiple blocks of memory at once when initialized to 0. The Free () function is used to dynamically delete. Assigned memory.
References:
- https://en.wikiversity.org/wiki/C_Programming/Memory_Management
- https://www.w3schools.in/c-tutorial/memory-management/
- https://www.tutorialcup.com/cprogramming/memory-management.htm
- https://www.guru99.com/c-dynamic-memory-allocation.html
What is Memory Management in C Language?
What is Object-Oriented Design
Why c is called procedure-oriented programming language?
What is Access Modifiers in C++
Classes and Objects in C++
What is Polymorphism in C++
Modern C++ Basics Part -4
What is C programming?
What is Inheritance in C++:
Advantages of C++ −