The ability of one class to derive properties and properties of another class is called inheritance. Inheritance is the most important characteristics of OOP.
What is child class?
A class that inherits properties from another class is called a child class and is also called a derived class or subclass.
What is parent class?
Classes whose properties are inherited by subclass are called parent class, superclass, or base class.
Example of Derived and Base Class:
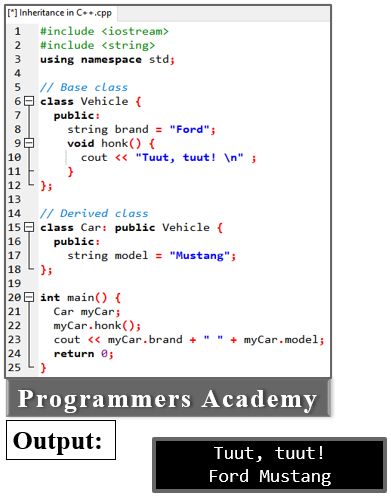
Syntax of Inheritance:
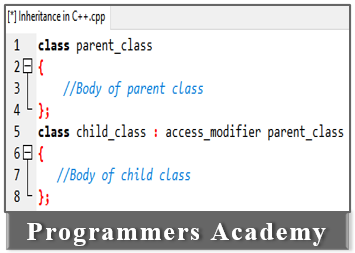
Inheritance Example:
In the program below, there are two classes, Teacher and MathTeacher. The MathTeacher class inherits from the Teacher class. In other words, Teacher is a class for parents and MathTeacher is a class for children. The child class can use the collegeName property of the parent class.
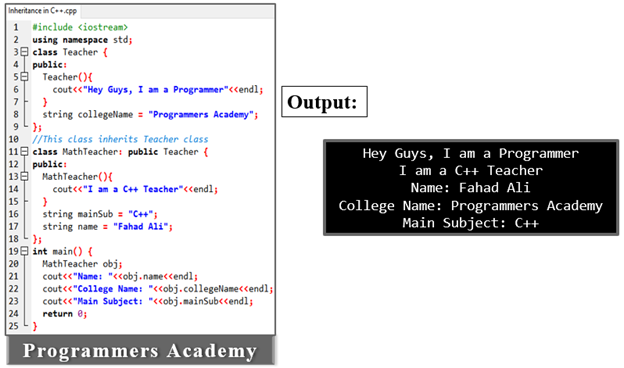
Modes of Inheritance:
- Public mode: By deriving a subclass from a public base class. The public members of the base class are made public in the derived class, and the protected members of the base class are protected in the derived class.
- Protected mode: By deriving a subclass from a protected base class. So both the public and protected members of the base class are protected by the derived class.
- Private mode: By deriving a subclass from a private base class. So both the public and protected members of the base class are private in the derived class.
Note: The derived class cannot directly access the private members of the base class, but the protected members can be directly accessed. For example, classes B, C, and D contain all variables x, y, and z in the following example. It is an access problem.
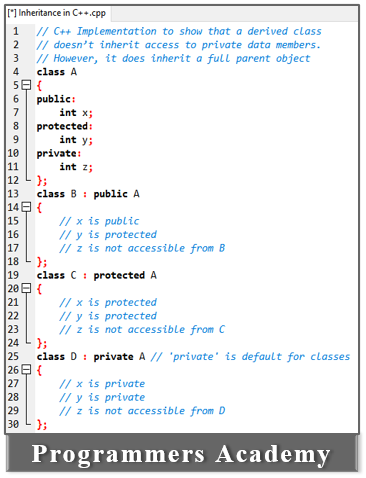
The below table summarizes the above three modes and shows access specifiers for base class members of subclass when derived in public, protected, and private modes.
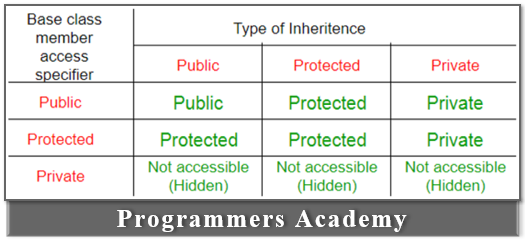
Types of Inheritance:
1) Single inheritance
2) Multilevel inheritance
3) Multiple inheritance
4) Hierarchical inheritance
5) Hybrid inheritance
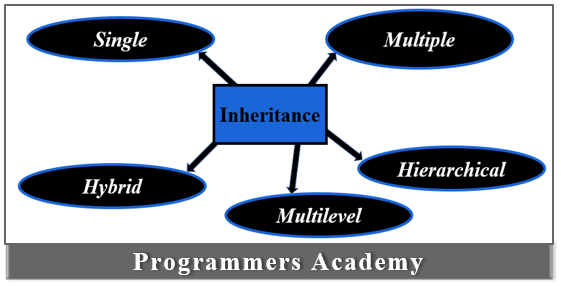
1. Single Inheritance:
In single inheritance, Classes can only be inherited from one class. That is, a single base class inherits one subclass.

Syntax of Single Inheritance:
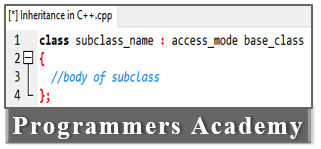
Example of Single Inheritance:
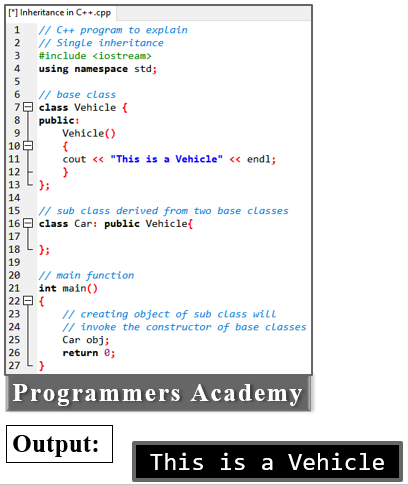
2. Multiple Inheritance:
Multiple inheritance is a feature of C ++ that allows a class to inherit from multiple classes. That is, a sub class is inherited from several base classes.
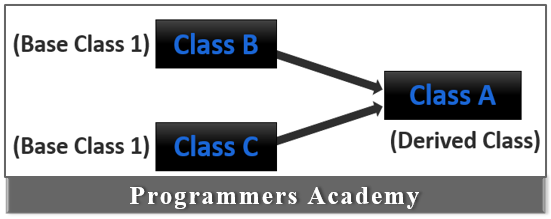
Syntax of Multiple Inheritance:
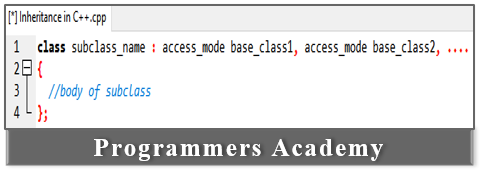
Here, the number of base classes must be separated by commas (“,”) to specify the access mode for all base classes.
Example of Multiple Inheritance:
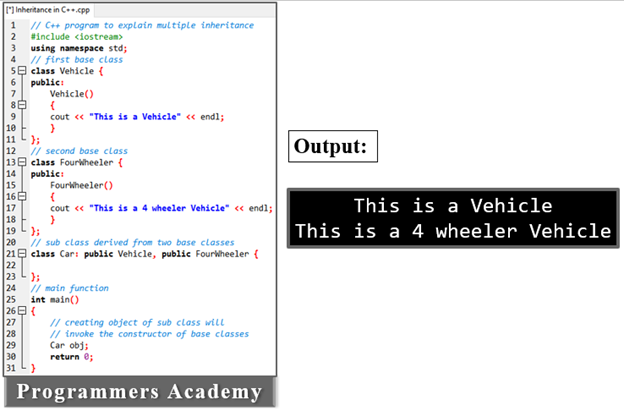
3. Multilevel Inheritance:
In this type of inheritance, the derived class is created from another derived class.
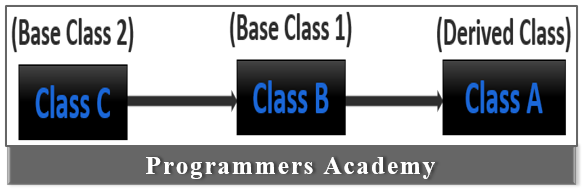
Example of Multilevel Inheritance:
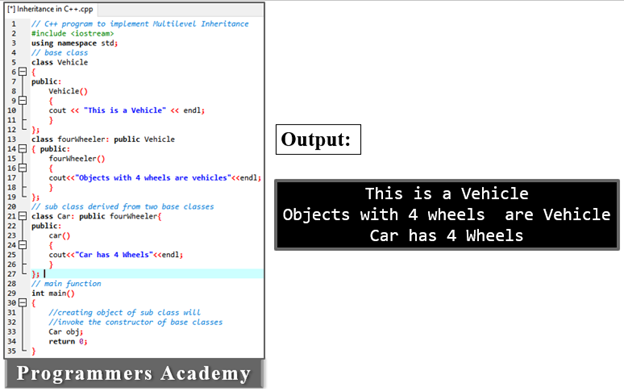
4. Hierarchical Inheritance:
In this type of inheritance, multiple subclasses inherit from a single base class. That is, multiple derived classes are created from a single base class.
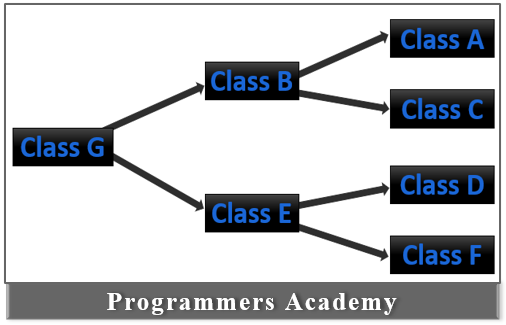
Example of Hierarchical Inheritance:
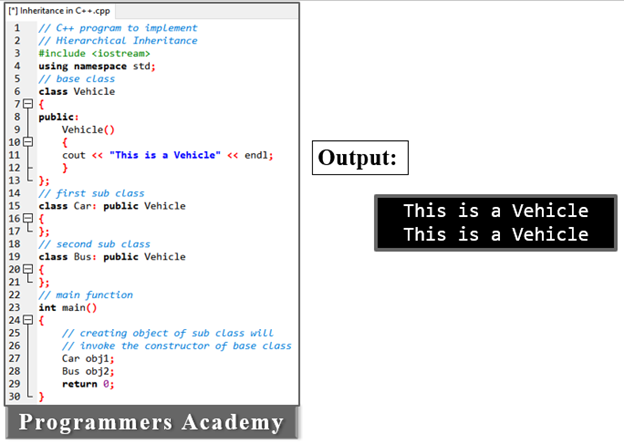
5. Hybrid (Virtual) Inheritance:
Hybrid inheritance is implemented by combining several types of inheritance. Example: a combination of hierarchical inheritance and multiple inheritance.
The following image shows a combination of hierarchical inheritance and multiple inheritance.
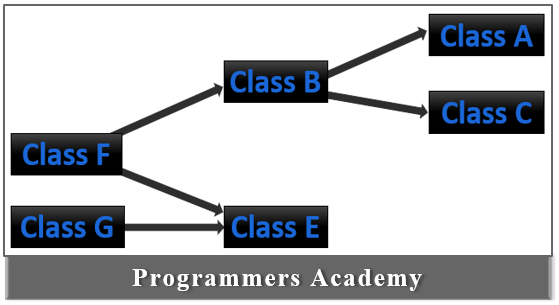
Example of Hybrid Inheritance:
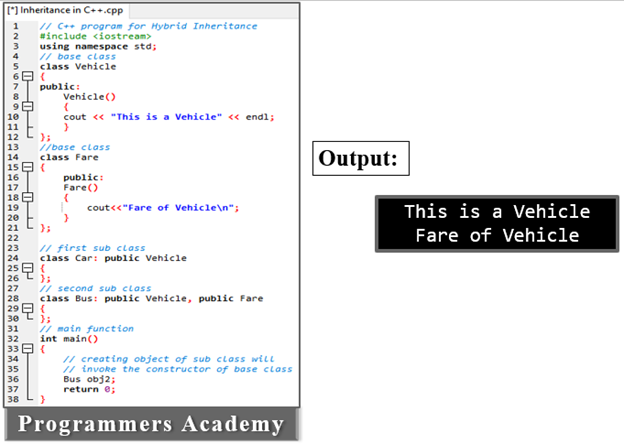
Advantages of Inheritance:
Inheritance allows you to inherit all the properties of a base class and access all the functionality of the inherited class. Implement code reuse.
- The main advantage of inheritance is that it helps you reuse your code. The code is defined only once and can be used multiple times.
- Heredity promotes reuse. If a class inherits or derives from another class, it can access all the functionality of the inherited class.
- Reuse improves reliability. The base class code has already been tested and debugged.
- Reuse existing code, reducing development and maintenance costs.
- Inheritance makes subclasses follow the standard interface.
- Inheritance reduces code redundancy and supports code extensibility.
- Inheritance makes it easy to create class libraries.
- Improves the structure of the readable program.
- The structure of the program is short, concise, and reliable.
- The code is easy to debug. Inheritance makes it easier for programs to catch errors.
- Inheritance gives you more flexibility in modifying your application code.
Disadvantages of Inheritance:
Improper use of inheritance or lack of knowledge about inheritance can lead to incorrect solutions.
- The main drawback of inheritance is that the two classes (base class and superclass) are tightly coupled. That is, the classes are interdependent.
- Inherited functions are slower than regular functions because they have indirection.
- The inappropriate use of inheritance can lead to an incorrect solution.
- In many cases, the data members of the base class will not be used, which can waste memory.
- Inheritance increases the link between the base class and the derived class. Changes to the base class affect all child classes.
- If you change the functionality of the base class, you must also make the change in the child class.
- If a superclass method is removed, it is very difficult to maintain the functionality of the child class that implements the superclass method.
Conclusion of Inheritance:
Inheritance is one of the most important components of object-oriented programming and is a hierarchy of inheritance classes. At this point, the notion that inheritance is used by a parent or base class through a derived class should be erased. We have already seen that C ++ supports both single and multiple inheritance. We have also seen three different types of inheritance. If you want the protected members of the base class to be the same as the protected members and the public members to be the same as the public members of the derived class, that is, you want to keep these two access specifiers in the derived class. But most of the time, programmers are interested in public inheritance. In general, it is a good idea not to exclude publicly accessible specifiers. One of the biggest benefits of inheritance is that access control specifiers allow derived classes to define their own members and redefine the members of the base class. This means that derived classes can override inherited properties and add new ones.
At the end of the inheritance conclusion, inheritance is just a convenience for programmers. If you need simple inheritance, try to avoid complex classes and overcome or minimize such situations. If you cannot avoid it and you really want to include multiple inheritance, you’d better avoid more complex situations. If you try to avoid all this and model the situation in the simplest way possible, nothing is simpler.
References:
- https://www.educative.io/edpresso/inheritance-in-cpp
- https://www.programiz.com/cpp-programming/inheritance
- https://www.w3schools.in/cplusplus-tutorial/inheritance/
- https://www.slideshare.net/AdilAslam4/inheritance-in-c-69290880