All C programs perform three main functions. That is, it takes the data as input, processes the data, and produces an output. The input refers to the acceptance of data and the output refers to the presentation of data. Input is generally accepted from the keyboard and output is displayed on the screen. I / O operations are the most common task in all programming languages.
Types of Input and Output Function:
In C, there are two main types of I / O functions …
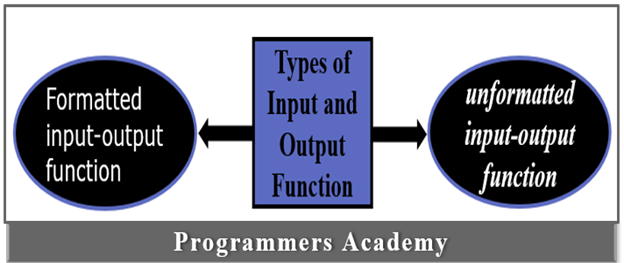
1. Formatted Input-Output Functions:
A formatted I / O function accepts or presents data in a particular format. The standard library consists of several functions that perform input and output operations. Of these functions, printf () and scanf () allow the user to format the I / O to the desired format. printf () and scanf () can be used to read any type of data (integers, real numbers, characters, etc.).
Types of Formatted Input-Output Function:
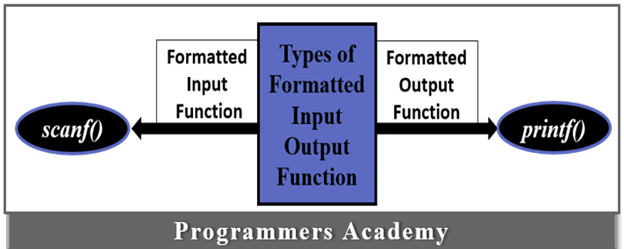
a. printf():
printf () is a formatted output function used to print information about the standard output unit. It is defined in the standard header file stdio.h. Therefore, to use printf (), you must include the stdio.h header file in your program.
Syntax of prinf():
printf(“format_string”, var1, var2, var3, …, varN);
For example:
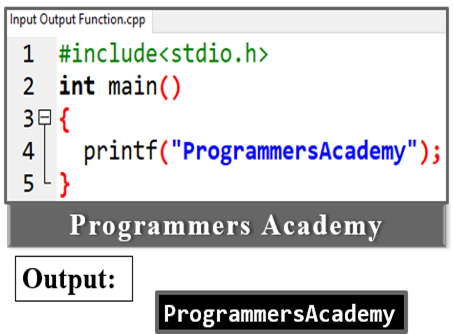
Here Code:
#include
int main()
{
printf("ProgrammersAcademy");
}
b. scanf():
scanf () is a formatted input function used to read formatted data from standard input devices, which automatically converts numeric information to integers and floating point numbers. It is defined in stdio.h.
Syntax of scanf():
scanf(“format_string”, &var1, &var2, &var3, …, &varN);
For example:
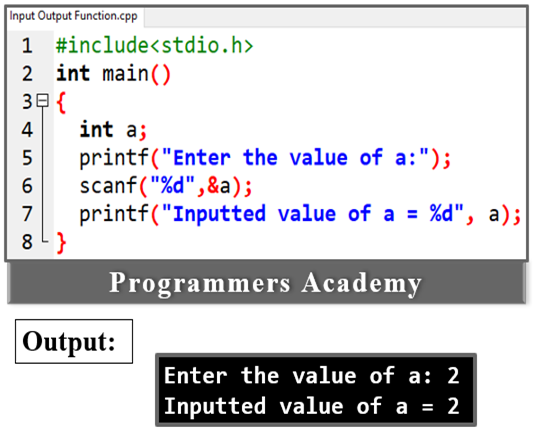
Here Code:
#include<stdio.h>
int main()
{
int a;
printf("Enter the value of a:");
scanf("%d",&a);
printf("Inputted value of a = %d", a);
}
2. Unformatted Input-Output Functions:
The unformatted I / O functions have no control over the data read and write format. These functions are the most basic form of input and output and cannot provide display input or output in the format that the user wants. Therefore, they are called raw I / O functions. Raw I / O functions are divided into two categories: character I / O functions and string I / O functions.
The character input function is used to read a character from the keyboard and the character output function is used to write a character on the screen. getch (), getche (), and getchar () are raw character input functions, and putch () and putchar () are raw character output functions.
Types of unformatted Input-Output Function:
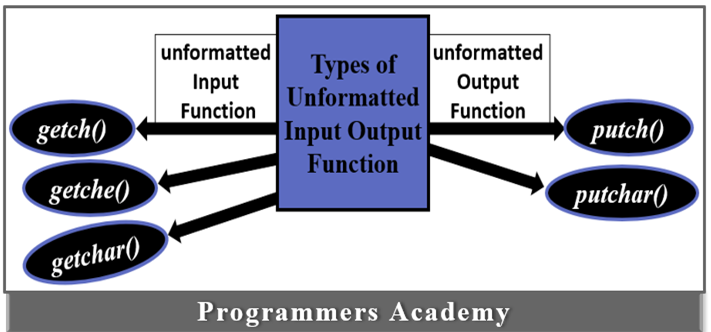
a. getch():
getch () is a character input function. This is a raw input function, which means that the user cannot read the input in its own format. Reads a character from the keyboard, but does not repeat the typed character and returns the typed character.
Syntax of getch():
character_variable = getch();
For example:
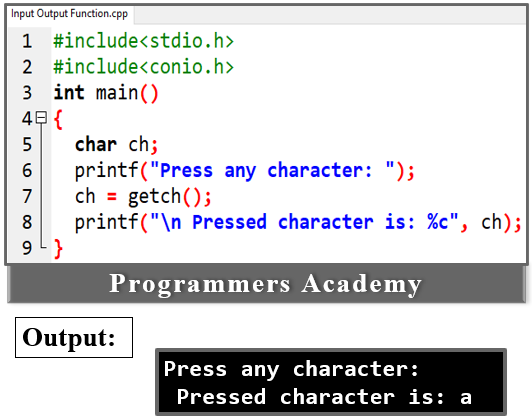
Here Code:
#include<stdio.h>
#include<conio.h>
int main()
{
char ch;
printf("Press any character: ");
ch = getch();
printf("\n Pressed character is: %c", ch);
}
b. getche():
Like getch (), getch () is a character input function. This is a raw input function, which means that the user cannot read the input in its own format. The difference between getch () and getche () is that getche () echoes the pressed character. getche () also returns the pressed character, like getch (). It is also defined in the header file conio.h.
Syntax of getche():
character_variable = getche();
For example:
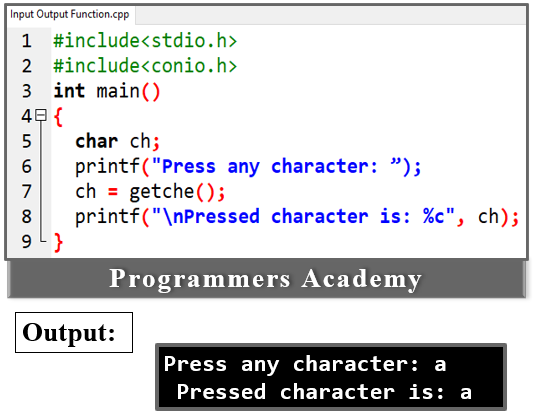
Here code:
#include<stdio.h>
#include<conio.h>
int main()
{
char ch;
printf("Press any character: ”);
ch = getche();
printf("\nPressed character is: %c", ch);
}
c. getchar():
getchar () is also a character input function. Reads a character and accepts the input until a carriage return is entered (that is, until the Enter key is pressed).
Syntax of getchar():
character_variable = getchar();
For example:
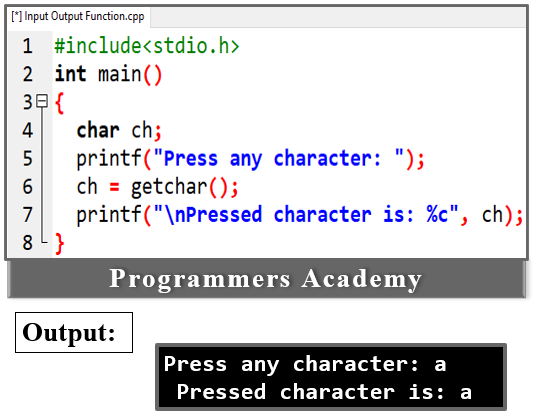
Here Code:
#include<stdio.h>
int main()
{
char ch;
printf("Press any character: ");
ch = getchar();
printf("\nPressed character is: %c", ch);
}
d. putch():
The putch () function is used to print characters on the screen at the current cursor position. Raw character output function.
Syntax of putch():
putch(character);
or
putch(character_variable);
For example:
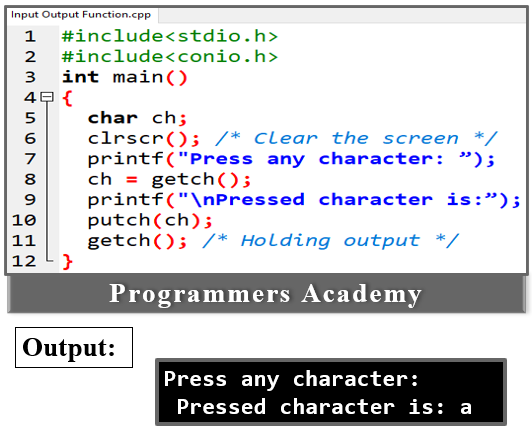
Here Code:
#include<stdio.h>
#include<conio.h>
int main()
{
char ch;
clrscr(); /* Clear the screen */
printf("Press any character: ”);
ch = getch();
printf("\nPressed character is:”);
putch(ch);
getch(); /* Holding output */
}
e. putchar():
The putchar () function is used to print a character on the screen at the current cursor position. Raw character output function.
Syntax of putchar():
putchar(character);
or
putchar(character_variable);
For example:
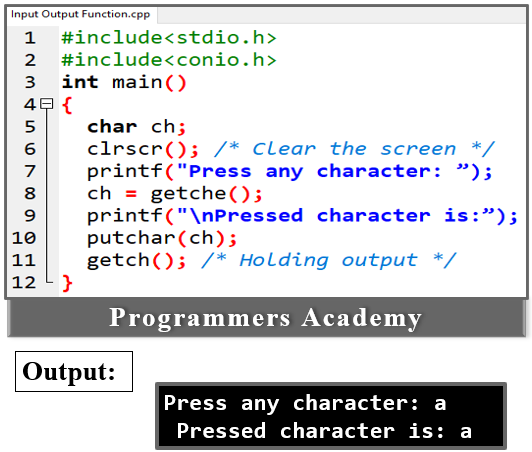
Here Code:
#include<stdio.h>
#include<conio.h>
int main()
{
char ch;
clrscr(); /* Clear the screen */
printf("Press any character: ”);
ch = getche();
printf("\nPressed character is:”);
putchar(ch);
getch(); /* Holding output */
}
In programming, a collection of characters is known as a string or array of characters. In C programming, there are several I / O functions that you can use to read and write strings. The most used are gets () and put (). get () is a raw input function for reading strings, and put () is a raw output function for writing strings.
f. gets():
gets () is a string input function. Read the keyboard string. Read the string until the Enter key is pressed.
Syntax of gets():
gets(string_variable);
For example:
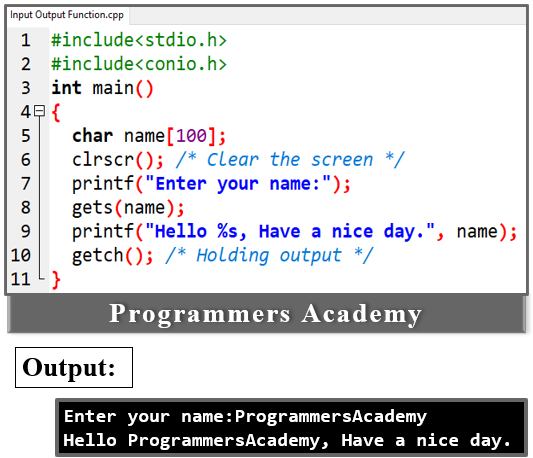
Here Code:
#include<stdio.h>
#include<conio.h>
int main()
{
char name[100];
clrscr(); /* Clear the screen */
printf("Enter your name:");
gets(name);
printf("Hello %s, Have a nice day.", name);
getch(); /* Holding output */
}
g. puts():
put () is a raw string output function. Type or print a string of characters on the screen.
Syntax of puts():
puts(string or string_variable);
For example:
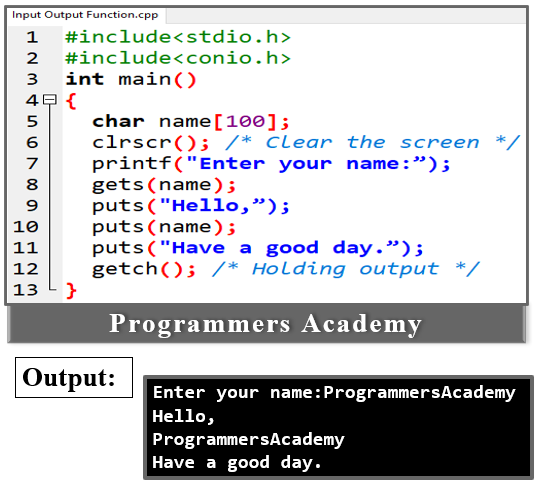
Here code:
#include<stdio.h>
#include<conio.h>
int main()
{
char name[100];
clrscr(); /* Clear the screen */
printf("Enter your name:”);
gets(name);
puts("Hello,”);
puts(name);
puts("Have a good day.”);
getch(); /* Holding output */
}
References:
- http://www.trytoprogram.com/c-programming-input-output-functions/
- https://tutorialink.com/c/io.c
- https://www.programiz.com/c-programming/c-input-output
- https://www.codesansar.com/c-programming/input-and-output-functions.htm
- https://www.studytonight.com/c/c-input-output-function.php
- https://www.w3schools.in/c-tutorial/input-output/