C Hello World! Example: Your First Program
Here,is a Hello World program in C
#include<stdio.h> //Pre-processor directive void main() //main function declaration { printf("Hello World"); //to output the string on a display getch (); //terminating function }
Here is the code explanation:
Pre-processor directive
#include is a pre-processor directive in ‘C.’
#include <stdio.h>, stdio is the library where the function printf is defined. printfis used for generating output. Before using this function, we have to first include the required file, also known as a header file (.h).
You can also create your own functions, group them in header files and declare them at the top of the program to use them. To include a file in a program, use pre-processor directive
#include <file-name>.h
File-name is the name of a file in which the functions are stored. Pre-processor directives are always placed at the beginning of the program.
The main function
The main function is a part of every ‘C’ program. We can represent the main function in various forms, such as:
- main()
- int main()
- void main()
- main(void)
- void main(void)
- int main(void)
The empty parentheses indicate that this function does not take any argument, value or a parameter. You can also represent this explicitly by placing the keyword void inside the parentheses. The keyword void means the function does not return any value, in this case, the last statement is always getch ().
#include<stdio.h> //Pre-processor directive int main() //main function declaration { printf("Hello World"); //to output the string on a display return 0; //terminating function }
In the above example, the keyword int means the function will return an integer value. In this case, the last statement should always return 0.
The source code
After the main function has been declared, we have to specify the opening and closing parentheses. Curly brackets { }, indicate the starting and end of a program. These brackets must be always put after the main function. All the program code is written inside these brackets, such as declarative and executable part.
The printf function generates the output by passing the text “Hello World!”
The semicolon ; determines the end of the statement. In C, each statement must end with a semicolon.
So we have successfully installed the compiler and now can begin working in ‘C.’ We will write a simple program that will say hello to us. Let’s start.
How to run C Program
Step 1) Create a new Project
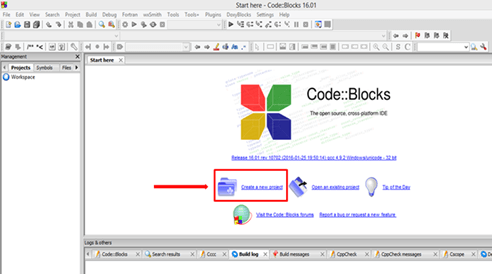
Step 2) In the pop-up,
- Select File
- Choose the “C/C++ Source”
- Click “Go.”
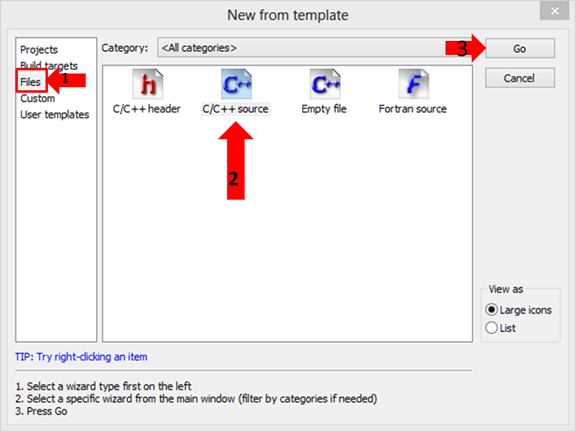
Step 3) Continue, by clicking on “Next.”
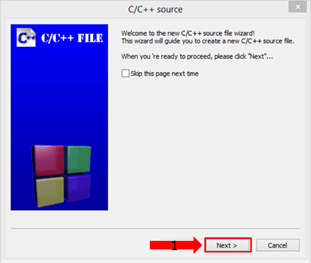
Step 4) To create the new file ,select a “C” file then click on “Next” button to continue.
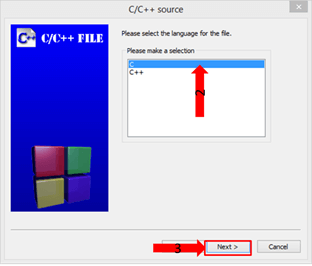
Step 5) Set the file path by clicking the “…” button, the explorer window permits to create the C file.
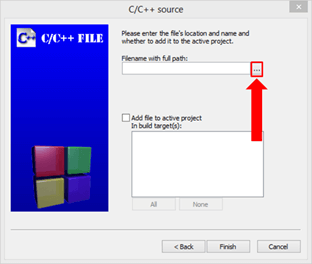
Step 6) Select the path of your new C File then its name which has .c extension and save it.
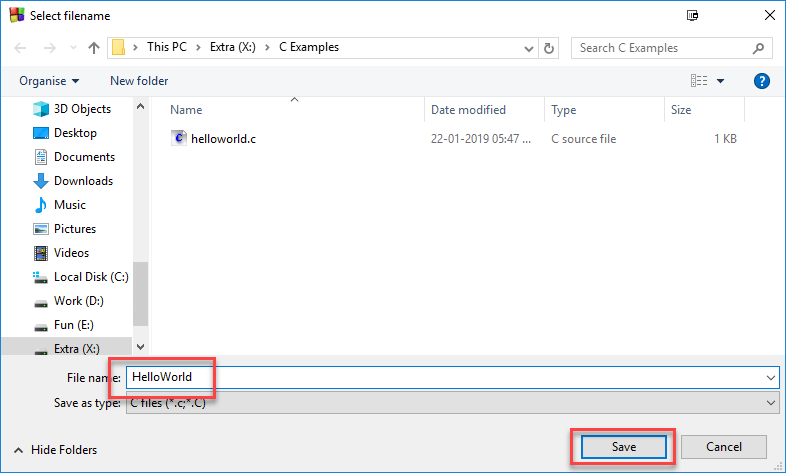
Step 7) Finally, to confirm the C file creation click “Finish.”
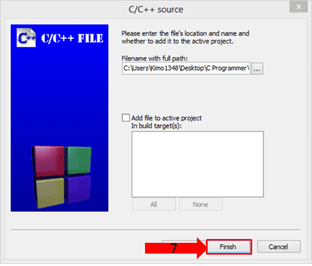
Step 8) Enter the code, save it and compile it by clicking on the “Build & Run “button.
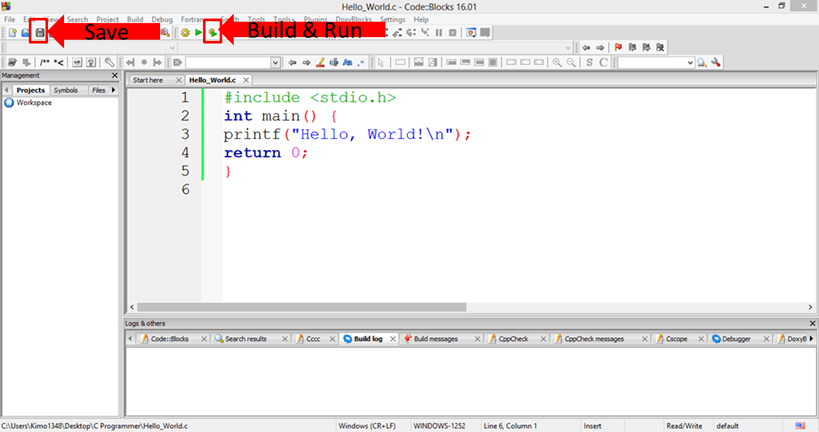
Here is the result:
Hello, World!
Summary
- The main function is a mandatory part of every ‘C’ program.
- To use the functionality of a header file, we have to include the file at the beginning of our program.
- Every ‘C’ program follows a basic structure.