In this article, we will explore the concept of classes and objects in C Plus Plus programming language. Classes and objects are fundamental building blocks of object-oriented programming (OOP) and play a crucial role in organizing and modeling real-world entities in software development.
All About Class In C++ Language
What is a Class?
C ++ classes combine data and methods to manipulate data into one. The class also determines the format of the object. The data and methods contained in a class are called class members. Classes are user-defined data types. Use an instance of the class to access the members of the class. The class can be viewed as a blueprint of the object.
Class Declaration:
In C +, classes are defined using the class keyword. This must be followed by the name of the class. Then the body of the class is added between the braces {}.
Class Syntax:

- class-name is the name you assign to the class.
- Data is class data and is generally declared as a variable.
- The function is a class function.
Create a Class
To create class, use class
keyword:
For example:
Create a class called “MyClass”:
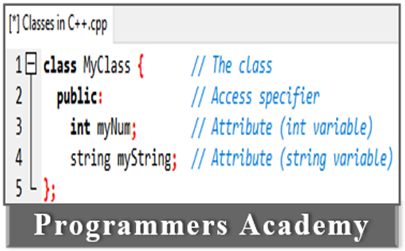
[sc name=”compiler”]
Example Explained:
- The class keyword used to create class called MyClass.
- The public keyword is access specifier that specifies the members of class (attributes and methods) so that they accessed from outside class. We will talk more access specifiers later.
- In class, an integer variable myNum and string variable myString. When variables are declared in class, they are known as attributes.
- Finally, end class definition with semicolon ; .
What is a Public Class?
The public keyword, on the other hand, exposes the data / function. These can be accessed from outside the classroom.
Public Class Example:

[sc name=”compiler”]
What is a Private Class?
Defining a function or class with the private keyword makes it private. It only accessed within class.
Private Class Example:
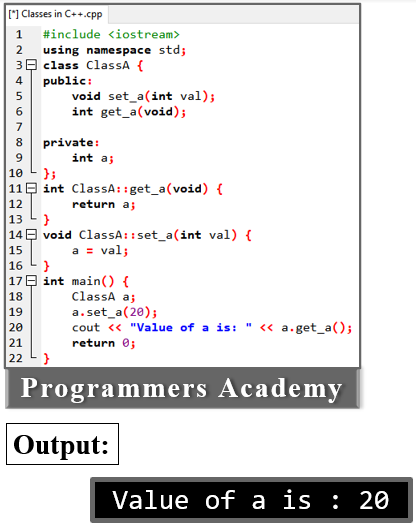
[sc name=”compiler”]
What is a Protected Class?
Protected ones have an advantage over those marked private. They can be accessed from their definition class functions. Also, it can be accessed from derived classes.
Protected Class Example:

[sc name=”compiler”]
Member Functions in Classes:
Two ways to define member function:
- Inside class definition
- Outside class definition
The following example defines a function in a class and names it “myMethod”.
Note: Access the method the same way you access the attribute. Create a class object and use dot (.) Syntax:
Learn More About : Access Modifiers in C Plus Plus
Inside Example:

[sc name=”compiler”]
To define a function outside of the class definition, you must declare the function inside the class and then define it outside the class. This is done by specifying the class name, the scope resolution :: operator, and the function name.
Outside Example:
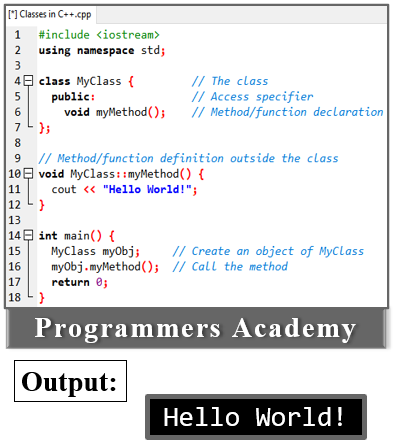
[sc name=”compiler”]
All About Object In C++ Language
What is a Object?
The object is an instance of the class. Memory is not allocated when the class is defined, but memory is allocated when an instance is created (that is, the object is created).
Object Declaration:
Once the class is defined, only the specifications of the object are defined. No memory or storage is allocated. You must create an object to use the data and access functions defined in the class.
Object Syntax:
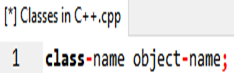
- class-name is the name of the class from which the object is created.
- object-name is the name assigned to the new object.
Process to creating object from class is known as instantiation.
Create an Object
In C ++, objects are created from classes. You have already created a class called MyClass and can use it to create objects.
To create a MyClass object, specify the name of the class followed by the name of the object.
To access the class attributes (myNum and myString), use the syntax of periods (.) On the object.
For example:
Create object called “myObj
“ and access attributes:
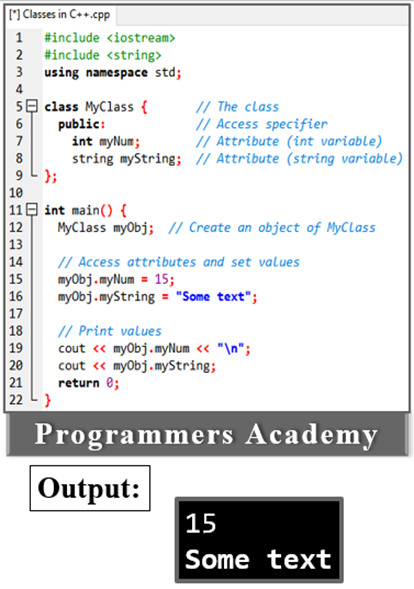
[sc name=”compiler”]
Multiple Objects:
You create multiple objects in one class:
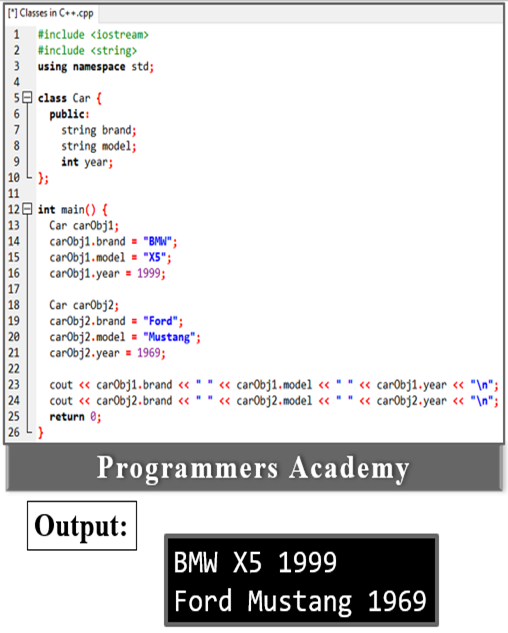
[sc name=”compiler”]
Accessing data members and member functions used in Classes and Objects:
You can access the data members and the functions of the class members in the object using the period operator (“.”). For example, if the object is called obj and you want to access a member function called printName (), you would type obj.printName ().
Accessing Data Members:
You can access public data members in the same way, but you cannot access private data members directly from objects. Access to a data member depends solely on the access control of that data member.
This access control is provided by the C ++ access modifiers. public, private and protected( This is three access Modifier)