[ad_1]
Origin Story
It began just a few years in the past when members of certainly one of my groups requested,
“what sample ought to we undertake for dependency injection (DI)”?
The crew’s stack was Typescript on Node.js, not one I used to be terribly aware of, so I
inspired them to work it out for themselves. I used to be upset to be taught
a while later that crew had determined, in impact, to not determine, leaving
behind a plethora of patterns for wiring modules collectively. Some builders
used manufacturing unit strategies, others guide dependency injection in root modules,
and a few objects in school constructors.
The outcomes had been lower than excellent: a hodgepodge of object-oriented and
purposeful patterns assembled in numerous methods, every requiring a really
totally different method to testing. Some modules had been unit testable, others
lacked entry factors for testing, so easy logic required advanced HTTP-aware
scaffolding to train fundamental performance. Most critically, modifications in
one a part of the codebase typically brought about damaged contracts in unrelated areas.
Some modules had been interdependent throughout namespaces; others had utterly flat collections of modules with
no distinction between subdomains.
With the advantage of hindsight, I continued to suppose
about that unique resolution: what DI sample ought to we’ve got picked.
Finally I got here to a conclusion: that was the incorrect query.
Dependency injection is a method, not an finish
On reflection, I ought to have guided the crew in the direction of asking a distinct
query: what are the specified qualities of our codebase, and what
approaches ought to we use to attain them? I want I had advocated for the
following:
- discrete modules with minimal incidental coupling, even at the price of some duplicate
varieties - enterprise logic that’s stored from intermingling with code that manages the transport,
like HTTP handlers or GraphQL resolvers - enterprise logic exams that aren’t transport-aware or have advanced
scaffolding - exams that don’t break when new fields are added to varieties
- only a few varieties uncovered outdoors of their modules, and even fewer varieties uncovered
outdoors of the directories they inhabit.
Over the previous few years, I’ve settled on an method that leads a
developer who adopts it towards these qualities. Having come from a
Take a look at-Pushed Growth (TDD) background, I naturally begin there.
TDD encourages incrementalism however I wished to go even additional,
so I’ve taken a minimalist “function-first” method to module composition.
Quite than persevering with to explain the method, I’ll exhibit it.
What follows is an instance net service constructed on a comparatively easy
structure whereby a controller module calls area logic which in flip
calls repository capabilities within the persistence layer.
The issue description
Think about a person story that appears one thing like this:
As a registered person of RateMyMeal and a would-be restaurant patron who
does not know what’s out there, I want to be supplied with a ranked
set of beneficial eating places in my area based mostly on different patron scores.
Acceptance Standards
- The restaurant listing is ranked from essentially the most to the least
beneficial. - The score course of contains the next potential score
ranges: - glorious (2)
- above common (1)
- common (0)
- beneath common (-1)
- horrible (-2).
- The general score is the sum of all particular person scores.
- Customers thought of “trusted” get a 4X multiplier on their
score. - The person should specify a metropolis to restrict the scope of the returned
restaurant.
Constructing an answer
I’ve been tasked with constructing a REST service utilizing Typescript,
Node.js, and PostgreSQL. I begin by constructing a really coarse integration
as a strolling skeleton that defines the
boundaries of the issue I want to resolve. This take a look at makes use of as a lot of
the underlying infrastructure as attainable. If I exploit any stubs, it is
for third-party cloud suppliers or different providers that may’t be run
domestically. Even then, I exploit server stubs, so I can use actual SDKs or
community shoppers. This turns into my acceptance take a look at for the duty at hand,
protecting me targeted. I’ll solely cowl one “glad path” that workout routines the
fundamental performance because the take a look at will likely be time-consuming to construct
robustly. I am going to discover more cost effective methods to check edge circumstances. For the sake of
the article, I assume that I’ve a skeletal database construction that I can
modify if required.
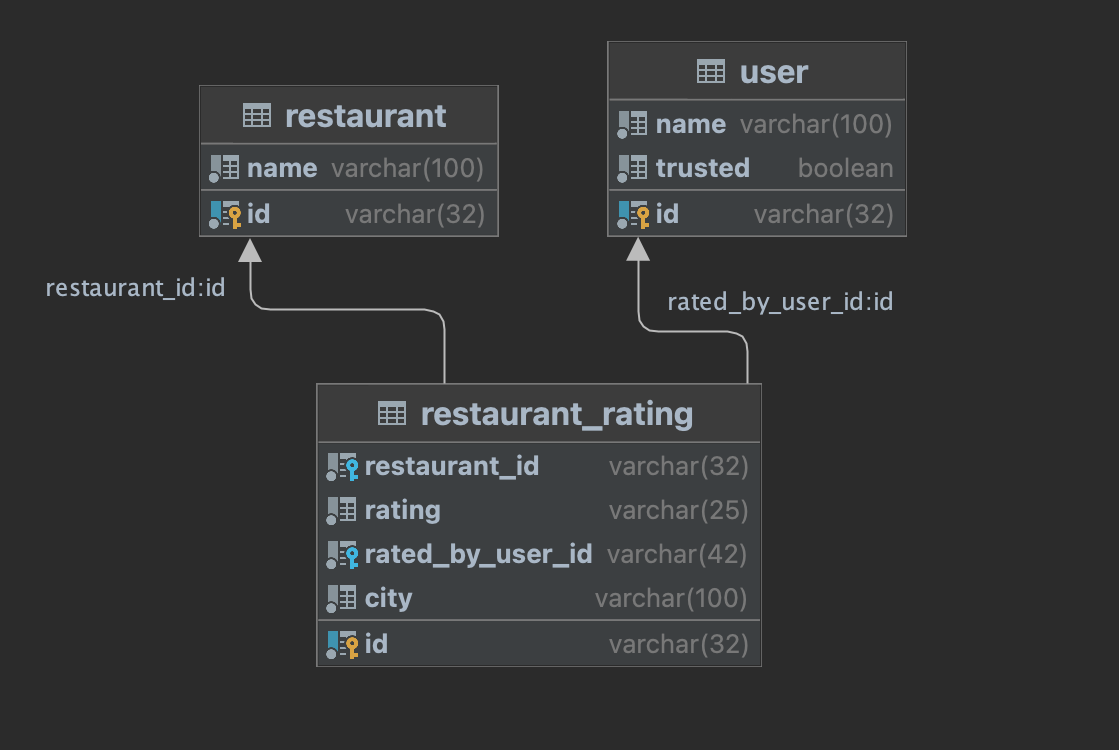
Assessments usually have a given/when/then
construction: a set of
given circumstances, a collaborating motion, and a verified end result. I choose to
begin at when/then
and again into the given
to assist me focus the issue I am making an attempt to unravel.
“When I name my advice endpoint, then I anticipate to get an OK response
and a payload with the top-rated eating places based mostly on our scores
algorithm”. In code that may very well be:
take a look at/e2e.integration.spec.ts…
describe("the eating places endpoint", () => { it("ranks by the advice heuristic", async () => { const response = await axios.get<ResponsePayload>( ➀ "http://localhost:3000/vancouverbc/eating places/beneficial", { timeout: 1000 }, ); anticipate(response.standing).toEqual(200); const knowledge = response.knowledge; const returnRestaurants = knowledge.eating places.map(r => r.id); anticipate(returnRestaurants).toEqual(["cafegloucesterid", "burgerkingid"]); ➁ }); }); kind ResponsePayload = { eating places: { id: string; identify: string }[]; };
There are a few particulars value calling out:
Axios
is the HTTP shopper library I’ve chosen to make use of.
The Axiosget
operate takes a sort argument
(ResponsePayload
) that defines the anticipated construction of
the response knowledge. The compiler will make it possible for all makes use of of
response.knowledge
conform to that kind, nevertheless, this test can
solely happen at compile-time, so can not assure the HTTP response physique
really incorporates that construction. My assertions might want to do
that.- Quite than checking the whole contents of the returned eating places,
I solely test their ids. This small element is deliberate. If I test the
contents of the whole object, my take a look at turns into fragile, breaking if I
add a brand new discipline. I wish to write a take a look at that may accommodate the pure
evolution of my code whereas on the similar time verifying the precise situation
I am enthusiastic about: the order of the restaurant itemizing.
With out my given
circumstances, this take a look at is not very invaluable, so I add them subsequent.
take a look at/e2e.integration.spec.ts…
describe("the eating places endpoint", () => { let app: Server | undefined; let database: Database | undefined; const customers = [ { id: "u1", name: "User1", trusted: true }, { id: "u2", name: "User2", trusted: false }, { id: "u3", name: "User3", trusted: false }, ]; const eating places = [ { id: "cafegloucesterid", name: "Cafe Gloucester" }, { id: "burgerkingid", name: "Burger King" }, ]; const ratingsByUser = [ ["rating1", users[0], eating places[0], "EXCELLENT"], ["rating2", users[1], eating places[0], "TERRIBLE"], ["rating3", users[2], eating places[0], "AVERAGE"], ["rating4", users[2], eating places[1], "ABOVE_AVERAGE"], ]; beforeEach(async () => { database = await DB.begin(); const shopper = database.getClient(); await shopper.join(); strive { // GIVEN // These capabilities do not exist but, however I am going to add them shortly for (const person of customers) { await createUser(person, shopper); } for (const restaurant of eating places) { await createRestaurant(restaurant, shopper); } for (const score of ratingsByUser) { await createRatingByUserForRestaurant(score, shopper); } } lastly { await shopper.finish(); } app = await server.begin(() => Promise.resolve({ serverPort: 3000, ratingsDB: { ...DB.connectionConfiguration, port: database?.getPort(), }, }), ); }); afterEach(async () => { await server.cease(); await database?.cease(); }); it("ranks by the advice heuristic", async () => { // .. snip
My given
circumstances are carried out within the beforeEach
operate.
accommodates the addition of extra exams ought to
beforeEach
I want to make the most of the identical setup scaffold and retains the pre-conditions
cleanly unbiased of the remainder of the take a look at. You may discover a variety of
await
calls. Years of expertise with reactive platforms
like Node.js have taught me to outline asynchronous contracts for all
however essentially the most straight-forward capabilities.
Something that finally ends up IO-bound, like a database name or file learn,
must be asynchronous and synchronous implementations are very straightforward to
wrap in a Promise, if crucial. Against this, selecting a synchronous
contract, then discovering it must be async is a a lot uglier drawback to
resolve, as we’ll see later.
I’ve deliberately deferred creating specific varieties for the customers and
eating places, acknowledging I do not know what they appear like but.
With Typescript’s structural typing, I can proceed to defer creating that
definition and nonetheless get the advantage of type-safety as my module APIs
start to solidify. As we’ll see later, this can be a crucial means by which
modules could be stored decoupled.
At this level, I’ve a shell of a take a look at with take a look at dependencies
lacking. The subsequent stage is to flesh out these dependencies by first constructing
stub capabilities to get the take a look at to compile after which implementing these helper
capabilities. That may be a non-trivial quantity of labor, however it’s additionally extremely
contextual and out of the scope of this text. Suffice it to say that it
will usually encompass:
- beginning up dependent providers, equivalent to databases. I usually use testcontainers to run dockerized providers, however these may
even be community fakes or in-memory parts, no matter you like. - fill within the
create...
capabilities to pre-construct the entities required for
the take a look at. Within the case of this instance, these are SQLINSERT
s. - begin up the service itself, at this level a easy stub. We’ll dig a
little extra into the service initialization because it’s germaine to the
dialogue of composition.
In case you are enthusiastic about how the take a look at dependencies are initialized, you’ll be able to
see the outcomes within the GitHub repo.
Earlier than shifting on, I run the take a look at to ensure it fails as I’d
anticipate. As a result of I’ve not but carried out my service
begin
, I anticipate to obtain a connection refused error when
making my http request. With that confirmed, I disable my large integration
take a look at, since it is not going to cross for some time, and commit.
On to the controller
I usually construct from the skin in, so my subsequent step is to
deal with the principle HTTP dealing with operate. First, I am going to construct a controller
unit take a look at. I begin with one thing that ensures an empty 200
response with anticipated headers:
take a look at/restaurantRatings/controller.spec.ts…
describe("the scores controller", () => {
it("offers a JSON response with scores", async () => {
const ratingsHandler: Handler = controller.createTopRatedHandler();
const request = stubRequest();
const response = stubResponse();
await ratingsHandler(request, response, () => {});
anticipate(response.statusCode).toEqual(200);
anticipate(response.getHeader("content-type")).toEqual("utility/json");
anticipate(response.getSentBody()).toEqual({});
});
});
I’ve already began to do some design work that may end in
the extremely decoupled modules I promised. Many of the code is pretty
typical take a look at scaffolding, however should you look intently on the highlighted operate
name it would strike you as uncommon.
This small element is step one towards
partial utility,
or capabilities returning capabilities with context. Within the coming paragraphs,
I am going to exhibit the way it turns into the inspiration upon which the compositional method is constructed.
Subsequent, I construct out the stub of the unit underneath take a look at, this time the controller, and
run it to make sure my take a look at is working as anticipated:
src/restaurantRatings/controller.ts…
export const createTopRatedHandler = () => { return async (request: Request, response: Response) => {}; };
My take a look at expects a 200, however I get no calls to standing
, so the
take a look at fails. A minor tweak to my stub it is passing:
src/restaurantRatings/controller.ts…
export const createTopRatedHandler = () => { return async (request: Request, response: Response) => { response.standing(200).contentType("utility/json").ship({}); }; };
I commit and transfer on to fleshing out the take a look at for the anticipated payload. I
do not but know precisely how I’ll deal with the info entry or
algorithmic a part of this utility, however I do know that I want to
delegate, leaving this module to nothing however translate between the HTTP protocol
and the area. I additionally know what I need from the delegate. Particularly, I
need it to load the top-rated eating places, no matter they’re and wherever
they arrive from, so I create a “dependencies” stub that has a operate to
return the highest eating places. This turns into a parameter in my manufacturing unit operate.
take a look at/restaurantRatings/controller.spec.ts…
kind Restaurant = { id: string }; kind RestaurantResponseBody = { eating places: Restaurant[] }; const vancouverRestaurants = [ { id: "cafegloucesterid", name: "Cafe Gloucester", }, { id: "baravignonid", name: "Bar Avignon", }, ]; const topRestaurants = [ { city: "vancouverbc", restaurants: vancouverRestaurants, }, ]; const dependenciesStub = { getTopRestaurants: (metropolis: string) => { const eating places = topRestaurants .filter(eating places => { return eating places.metropolis == metropolis; }) .flatMap(r => r.eating places); return Promise.resolve(eating places); }, }; const ratingsHandler: Handler = controller.createTopRatedHandler(dependenciesStub); const request = stubRequest().withParams({ metropolis: "vancouverbc" }); const response = stubResponse(); await ratingsHandler(request, response, () => {}); anticipate(response.statusCode).toEqual(200); anticipate(response.getHeader("content-type")).toEqual("utility/json"); const despatched = response.getSentBody() as RestaurantResponseBody; anticipate(despatched.eating places).toEqual([ vancouverRestaurants[0], vancouverRestaurants[1], ]);
With so little data on how the getTopRestaurants
operate is carried out,
how do I stub it? I do know sufficient to design a fundamental shopper view of the contract I’ve
created implicitly in my dependencies stub: a easy unbound operate that
asynchronously returns a set of Eating places. This contract is perhaps
fulfilled by a easy static operate, a technique on an object occasion, or
a stub, as within the take a look at above. This module does not know, does not
care, and does not need to. It’s uncovered to the minimal it must do its
job, nothing extra.
src/restaurantRatings/controller.ts…
interface Restaurant { id: string; identify: string; } interface Dependencies { getTopRestaurants(metropolis: string): Promise<Restaurant[]>; } export const createTopRatedHandler = (dependencies: Dependencies) => { const { getTopRestaurants } = dependencies; return async (request: Request, response: Response) => { const metropolis = request.params["city"] response.contentType("utility/json"); const eating places = await getTopRestaurants(metropolis); response.standing(200).ship({ eating places }); }; };
For individuals who like to visualise these items, we are able to visualize the manufacturing
code as far as the handler operate that requires one thing that
implements the getTopRatedRestaurants
interface utilizing
a ball and socket notation.
The exams create this operate and a stub for the required
operate. I can present this through the use of a distinct color for the exams, and
the socket notation to indicate implementation of an interface.
This controller
module is brittle at this level, so I am going to have to
flesh out my exams to cowl various code paths and edge circumstances, however that is a bit past
the scope of the article. In the event you’re enthusiastic about seeing a extra thorough take a look at and the ensuing controller module, each can be found in
the GitHub repo.
Digging into the area
At this stage, I’ve a controller that requires a operate that does not exist. My
subsequent step is to supply a module that may fulfill the getTopRestaurants
contract. I am going to begin that course of by writing an enormous clumsy unit take a look at and
refactor it for readability later. It is just at this level I begin considering
about methods to implement the contract I’ve beforehand established. I’m going
again to my unique acceptance standards and attempt to minimally design my
module.
take a look at/restaurantRatings/topRated.spec.ts…
describe("The highest rated restaurant listing", () => { it("is calculated from our proprietary scores algorithm", async () => { const scores: RatingsByRestaurant[] = [ { restaurantId: "restaurant1", ratings: [ { rating: "EXCELLENT", }, ], }, { restaurantId: "restaurant2", scores: [ { rating: "AVERAGE", }, ], }, ]; const ratingsByCity = [ { city: "vancouverbc", ratings, }, ]; const findRatingsByRestaurantStub: (metropolis: string) => Promise< ➀ RatingsByRestaurant[] > = (metropolis: string) => { return Promise.resolve( ratingsByCity.filter(r => r.metropolis == metropolis).flatMap(r => r.scores), ); }; const calculateRatingForRestaurantStub: ( ➁ scores: RatingsByRestaurant, ) => quantity = scores => { // I do not know the way that is going to work, so I am going to use a dumb however predictable stub if (scores.restaurantId === "restaurant1") { return 10; } else if (scores.restaurantId == "restaurant2") { return 5; } else { throw new Error("Unknown restaurant"); } }; const dependencies = { ➂ findRatingsByRestaurant: findRatingsByRestaurantStub, calculateRatingForRestaurant: calculateRatingForRestaurantStub, }; const getTopRated: (metropolis: string) => Promise<Restaurant[]> = topRated.create(dependencies); const topRestaurants = await getTopRated("vancouverbc"); anticipate(topRestaurants.size).toEqual(2); anticipate(topRestaurants[0].id).toEqual("restaurant1"); anticipate(topRestaurants[1].id).toEqual("restaurant2"); }); }); interface Restaurant { id: string; } interface RatingsByRestaurant { ➃ restaurantId: string; scores: RestaurantRating[]; } interface RestaurantRating { score: Score; } export const score = { ➄ EXCELLENT: 2, ABOVE_AVERAGE: 1, AVERAGE: 0, BELOW_AVERAGE: -1, TERRIBLE: -2, } as const; export kind Score = keyof typeof score;
I’ve launched a variety of new ideas into the area at this level, so I am going to take them one by one:
- I want a “finder” that returns a set of scores for every restaurant. I am going to
begin by stubbing that out. - The acceptance standards present the algorithm that may drive the general score, however
I select to disregard that for now and say that, someway, this group of scores
will present the general restaurant score as a numeric worth. - For this module to operate it’s going to depend on two new ideas:
discovering the scores of a restaurant, and provided that set or scores,
producing an general score. I create one other “dependencies” interface that
contains the 2 stubbed capabilities with naive, predictable stub implementations
to maintain me shifting ahead. - The
RatingsByRestaurant
represents a set of
scores for a specific restaurant.RestaurantRating
is a
single such score. I outline them inside my take a look at to point the
intention of my contract. These varieties would possibly disappear in some unspecified time in the future, or I
would possibly promote them into manufacturing code. For now, it is a good reminder of
the place I am headed. Sorts are very low cost in a structurally-typed language
like Typescript, so the price of doing so could be very low. - I additionally want
score
, which, based on the ACs, consists of 5
values: “glorious (2), above common (1), common (0), beneath common (-1), horrible (-2)”.
This, too, I’ll seize inside the take a look at module, ready till the “final accountable second”
to determine whether or not to drag it into manufacturing code.
As soon as the essential construction of my take a look at is in place, I attempt to make it compile
with a minimalist implementation.
src/restaurantRatings/topRated.ts…
interface Dependencies {} export const create = (dependencies: Dependencies) => { ➀ return async (metropolis: string): Promise<Restaurant[]> => []; }; interface Restaurant { ➁ id: string; } export const score = { ➂ EXCELLENT: 2, ABOVE_AVERAGE: 1, AVERAGE: 0, BELOW_AVERAGE: -1, TERRIBLE: -2, } as const; export kind Score = keyof typeof score;
- Once more, I exploit my partially utilized operate
manufacturing unit sample, passing in dependencies and returning a operate. The take a look at
will fail, in fact, however seeing it fail in the best way I anticipate builds my confidence
that it’s sound. - As I start implementing the module underneath take a look at, I establish some
area objects that must be promoted to manufacturing code. Specifically, I
transfer the direct dependencies into the module underneath take a look at. Something that is not
a direct dependency, I go away the place it’s in take a look at code. - I additionally make one anticipatory transfer: I extract the
Score
kind into
manufacturing code. I really feel snug doing so as a result of it’s a common and specific area
idea. The values had been particularly known as out within the acceptance standards, which says to
me that couplings are much less more likely to be incidental.
Discover that the kinds I outline or transfer into the manufacturing code are not exported
from their modules. That may be a deliberate alternative, one I am going to talk about in additional depth later.
Suffice it to say, I’ve but to determine whether or not I need different modules binding to
these varieties, creating extra couplings that may show to be undesirable.
Now, I end the implementation of the getTopRated.ts
module.
src/restaurantRatings/topRated.ts…
interface Dependencies { ➀ findRatingsByRestaurant: (metropolis: string) => Promise<RatingsByRestaurant[]>; calculateRatingForRestaurant: (scores: RatingsByRestaurant) => quantity; } interface OverallRating { ➁ restaurantId: string; score: quantity; } interface RestaurantRating { ➂ score: Score; } interface RatingsByRestaurant { restaurantId: string; scores: RestaurantRating[]; } export const create = (dependencies: Dependencies) => { ➃ const calculateRatings = ( ratingsByRestaurant: RatingsByRestaurant[], calculateRatingForRestaurant: (scores: RatingsByRestaurant) => quantity, ): OverallRating[] => ratingsByRestaurant.map(scores => { return { restaurantId: scores.restaurantId, score: calculateRatingForRestaurant(scores), }; }); const getTopRestaurants = async (metropolis: string): Promise<Restaurant[]> => { const { findRatingsByRestaurant, calculateRatingForRestaurant } = dependencies; const ratingsByRestaurant = await findRatingsByRestaurant(metropolis); const overallRatings = calculateRatings( ratingsByRestaurant, calculateRatingForRestaurant, ); const toRestaurant = (r: OverallRating) => ({ id: r.restaurantId, }); return sortByOverallRating(overallRatings).map(r => { return toRestaurant(r); }); }; const sortByOverallRating = (overallRatings: OverallRating[]) => overallRatings.type((a, b) => b.score - a.score); return getTopRestaurants; }; //SNIP ..
Having carried out so, I’ve
- stuffed out the Dependencies kind I modeled in my unit take a look at
- launched the
OverallRating
kind to seize the area idea. This may very well be a
tuple of restaurant id and a quantity, however as I stated earlier, varieties are low cost and I consider
the extra readability simply justifies the minimal price. - extracted a few varieties from the take a look at that are actually direct dependencies of my
topRated
module - accomplished the easy logic of the first operate returned by the manufacturing unit.
The dependencies between the principle manufacturing code capabilities appear like
this
When together with the stubs supplied by the take a look at, it appears to be like ike this
With this implementation full (for now), I’ve a passing take a look at for my
principal area operate and one for my controller. They’re completely decoupled.
A lot so, actually, that I really feel the necessity to show to myself that they’ll
work collectively. It is time to begin composing the items and constructing towards a
bigger complete.
Starting to wire it up
At this level, I’ve a choice to make. If I am constructing one thing
comparatively straight-forward, I would select to dispense with a test-driven
method when integrating the modules, however on this case, I will proceed
down the TDD path for 2 causes:
- I wish to concentrate on the design of the integrations between modules, and writing a take a look at is a
good instrument for doing so. - There are nonetheless a number of modules to be carried out earlier than I can
use my unique acceptance take a look at as validation. If I wait to combine
them till then, I may need quite a bit to untangle if a few of my underlying
assumptions are flawed.
If my first acceptance take a look at is a boulder and my unit exams are pebbles,
then this primary integration take a look at could be a fist-sized rock: a chunky take a look at
exercising the decision path from the controller into the primary layer of
area capabilities, offering take a look at doubles for something past that layer. Not less than that’s how
it’s going to begin. I would proceed integrating subsequent layers of the
structure as I’m going. I additionally would possibly determine to throw the take a look at away if
it loses its utility or is getting in my approach.
After preliminary implementation, the take a look at will validate little greater than that
I’ve wired the routes appropriately, however will quickly cowl calls into
the area layer and validate that the responses are encoded as
anticipated.
take a look at/restaurantRatings/controller.integration.spec.ts…
describe("the controller prime rated handler", () => { it("delegates to the area prime rated logic", async () => { const returnedRestaurants = [ { id: "r1", name: "restaurant1" }, { id: "r2", name: "restaurant2" }, ]; const topRated = () => Promise.resolve(returnedRestaurants); const app = specific(); ratingsSubdomain.init( app, productionFactories.replaceFactoriesForTest({ topRatedCreate: () => topRated, }), ); const response = await request(app).get( "/vancouverbc/eating places/beneficial", ); anticipate(response.standing).toEqual(200); anticipate(response.get("content-type")).toBeDefined(); anticipate(response.get("content-type").toLowerCase()).toContain("json"); const payload = response.physique as RatedRestaurants; anticipate(payload.eating places).toBeDefined(); anticipate(payload.eating places.size).toEqual(2); anticipate(payload.eating places[0].id).toEqual("r1"); anticipate(payload.eating places[1].id).toEqual("r2"); }); }); interface RatedRestaurants { eating places: { id: string; identify: string }[]; }
These exams can get a bit of ugly since they rely closely on the net framework. Which
results in a second resolution I’ve made. I may use a framework like Jest or Sinon.js and
use module stubbing or spies that give me hooks into unreachable dependencies like
the topRated
module. I do not significantly wish to expose these in my API,
so utilizing testing framework trickery is perhaps justified. However on this case, I’ve determined to
present a extra typical entry level: the non-obligatory assortment of manufacturing unit
capabilities to override in my init()
operate. This offers me with the
entry level I want through the improvement course of. As I progress, I would determine I do not
want that hook anymore through which case, I am going to do away with it.
Subsequent, I write the code that assembles my modules.
src/restaurantRatings/index.ts…
export const init = ( specific: Categorical, factories: Factories = productionFactories, ) => { // TODO: Wire in a stub that matches the dependencies signature for now. // Substitute this as soon as we construct our extra dependencies. const topRatedDependencies = { findRatingsByRestaurant: () => { throw "NYI"; }, calculateRatingForRestaurant: () => { throw "NYI"; }, }; const getTopRestaurants = factories.topRatedCreate(topRatedDependencies); const handler = factories.handlerCreate({ getTopRestaurants, // TODO: <-- This line doesn't compile proper now. Why? }); specific.get("/:metropolis/eating places/beneficial", handler); }; interface Factories { topRatedCreate: typeof topRated.create; handlerCreate: typeof createTopRatedHandler; replaceFactoriesForTest: (replacements: Partial<Factories>) => Factories; } export const productionFactories: Factories = { handlerCreate: createTopRatedHandler, topRatedCreate: topRated.create, replaceFactoriesForTest: (replacements: Partial<Factories>): Factories => { return { ...productionFactories, ...replacements }; }, };
Generally I’ve a dependency for a module outlined however nothing to satisfy
that contract but. That’s completely tremendous. I can simply outline an implementation inline that
throws an exception as within the topRatedHandlerDependencies
object above.
Acceptance exams will fail however, at this stage, that’s as I’d anticipate.
Discovering and fixing an issue
The cautious observer will discover that there’s a compile error on the level the
is constructed as a result of I’ve a battle between two definitions:
topRatedHandler
- the illustration of the restaurant as understood by
controller.ts
- the restaurant as outlined in
topRated.ts
and returned
bygetTopRestaurants
.
The reason being easy: I’ve but so as to add a identify
discipline to the
kind in
RestauranttopRated.ts
. There’s a
trade-off right here. If I had a single kind representing a restaurant, fairly than one in every module,
I’d solely have so as to add identify
as soon as, and
each modules would compile with out extra modifications. Nonetheless,
I select to maintain the kinds separate, regardless that it creates
further template code. By sustaining two distinct varieties, one for every
layer of my utility, I am a lot much less more likely to couple these layers
unnecessarily. No, this isn’t very DRY, however I
am typically keen to threat some repetition to maintain the module contracts as
unbiased as attainable.
src/restaurantRatings/topRated.ts…
interface Restaurant { id: string; identify: string, } const toRestaurant = (r: OverallRating) => ({ id: r.restaurantId, // TODO: I put in a dummy worth to // begin and ensure our contract is being met // then we'll add extra to the testing identify: "", });
My extraordinarily naive answer will get the code compiling once more, permitting me to proceed on my
present work on the module. I am going to shortly add validation to my exams that be sure that the
identify
discipline is mapped accurately. Now with the take a look at passing, I transfer on to the
subsequent step, which is to supply a extra everlasting answer to the restaurant mapping.
Reaching out to the repository layer
Now, with the construction of my getTopRestaurants
operate extra or
much less in place and in want of a option to get the restaurant identify, I’ll fill out the
toRestaurant
operate to load the remainder of the Restaurant
knowledge.
Previously, earlier than adopting this extremely function-driven type of improvement, I in all probability would
have constructed a repository object interface or stub with a technique meant to load the
object. Now my inclination is to construct the minimal the I want: a
Restaurant
operate definition for loading the item with out making any assumptions concerning the
implementation. That may come later after I’m binding to that operate.
take a look at/restaurantRatings/topRated.spec.ts…
const restaurantsById = new Map<string, any>([
["restaurant1", { restaurantId: "restaurant1", name: "Restaurant 1" }],
["restaurant2", { restaurantId: "restaurant2", name: "Restaurant 2" }],
]);
const getRestaurantByIdStub = (id: string) => { ➀
return restaurantsById.get(id);
};
//SNIP...
const dependencies = { getRestaurantById: getRestaurantByIdStub, ➁ findRatingsByRestaurant: findRatingsByRestaurantStub, calculateRatingForRestaurant: calculateRatingForRestaurantStub, }; const getTopRated = topRated.create(dependencies); const topRestaurants = await getTopRated("vancouverbc"); anticipate(topRestaurants.size).toEqual(2); anticipate(topRestaurants[0].id).toEqual("restaurant1"); anticipate(topRestaurants[0].identify).toEqual("Restaurant 1"); ➂ anticipate(topRestaurants[1].id).toEqual("restaurant2"); anticipate(topRestaurants[1].identify).toEqual("Restaurant 2");
In my domain-level take a look at, I’ve launched:
- a stubbed finder for the
Restaurant
- an entry in my dependencies for that finder
- validation that the identify matches what was loaded from the
Restaurant
object.
As with earlier capabilities that load knowledge, the
getRestaurantById
returns a price wrapped in
Promise
. Though I proceed to play the little sport,
pretending that I do not know the way I’ll implement the
operate, I do know the Restaurant
is coming from an exterior
knowledge supply, so I’ll wish to load it asynchronously. That makes the
mapping code extra concerned.
src/restaurantRatings/topRated.ts…
const getTopRestaurants = async (metropolis: string): Promise<Restaurant[]> => { const { findRatingsByRestaurant, calculateRatingForRestaurant, getRestaurantById, } = dependencies; const toRestaurant = async (r: OverallRating) => { ➀ const restaurant = await getRestaurantById(r.restaurantId); return { id: r.restaurantId, identify: restaurant.identify, }; }; const ratingsByRestaurant = await findRatingsByRestaurant(metropolis); const overallRatings = calculateRatings( ratingsByRestaurant, calculateRatingForRestaurant, ); return Promise.all( ➁ sortByOverallRating(overallRatings).map(r => { return toRestaurant(r); }), ); };
- The complexity comes from the truth that
toRestaurant
is asynchronous - I can simply dealt with it within the calling code with
Promise.all()
.
I do not need every of those requests to dam,
or my IO-bound hundreds will run serially, delaying the whole person request, however I have to
block till all of the lookups are full. Fortunately, the Promise library
offers Promise.all
to break down a set of Guarantees
right into a single Promise containing a set.
With this transformation, the requests to lookup the restaurant exit in parallel. That is tremendous for
a prime 10 listing because the variety of concurrent requests is small. In an utility of any scale,
I’d in all probability restructure my service calls to load the identify
discipline through a database
be a part of and remove the additional name. If that possibility was not out there, for instance,
I used to be querying an exterior API, I would want to batch them by hand or use an async
pool as supplied by a third-party library like Tiny Async Pool
to handle the concurrency.
Once more, I replace by meeting module with a dummy implementation so it
all compiles, then begin on the code that fulfills my remaining
contracts.
src/restaurantRatings/index.ts…
export const init = ( specific: Categorical, factories: Factories = productionFactories, ) => { const topRatedDependencies = { findRatingsByRestaurant: () => { throw "NYI"; }, calculateRatingForRestaurant: () => { throw "NYI"; }, getRestaurantById: () => { throw "NYI"; }, }; const getTopRestaurants = factories.topRatedCreate(topRatedDependencies); const handler = factories.handlerCreate({ getTopRestaurants, }); specific.get("/:metropolis/eating places/beneficial", handler); };
The final mile: implementing area layer dependencies
With my controller and principal area module workflow in place, it is time to implement the
dependencies, particularly the database entry layer and the weighted score
algorithm.
This results in the next set of high-level capabilities and dependencies
For testing, I’ve the next association of stubs
For testing, all the weather are created by the take a look at code, however I
have not proven that within the diagram resulting from muddle.
The
course of for implementing these modules is follows the identical sample:
- implement a take a look at to drive out the essential design and a
Dependencies
kind if
one is critical - construct the essential logical circulate of the module, making the take a look at cross
- implement the module dependencies
- repeat.
I will not stroll by the whole course of once more since I’ve already exhibit the method.
The code for the modules working end-to-end is out there within the
repo. Some facets of the ultimate implementation require extra commentary.
By now, you would possibly anticipate my scores algorithm to be made out there through one more manufacturing unit carried out as a
partially utilized operate. This time I selected to write down a pure operate as an alternative.
src/restaurantRatings/ratingsAlgorithm.ts…
interface RestaurantRating { score: Score; ratedByUser: Person; } interface Person { id: string; isTrusted: boolean; } interface RatingsByRestaurant { restaurantId: string; scores: RestaurantRating[]; } export const calculateRatingForRestaurant = ( scores: RatingsByRestaurant, ): quantity => { const trustedMultiplier = (curr: RestaurantRating) => curr.ratedByUser.isTrusted ? 4 : 1; return scores.scores.scale back((prev, curr) => { return prev + score[curr.rating] * trustedMultiplier(curr); }, 0); };
I made this option to sign that this could all the time be
a easy, stateless calculation. Had I wished to depart a simple pathway
towards a extra advanced implementation, say one thing backed by knowledge science
mannequin parameterized per person, I’d have used the manufacturing unit sample once more.
Usually there is not a proper or incorrect reply. The design alternative offers a
path, so to talk, indicating how I anticipate the software program would possibly evolve.
I create extra inflexible code in areas that I do not suppose ought to
change whereas leaving extra flexibility within the areas I’ve much less confidence
within the path.
One other instance the place I “go away a path” is the choice to outline
one other RestaurantRating
kind in
ratingsAlgorithm.ts
. The kind is precisely the identical as
RestaurantRating
outlined in topRated.ts
. I
may take one other path right here:
- export
RestaurantRating
fromtopRated.ts
and reference it immediately inratingsAlgorithm.ts
or - issue
RestaurantRating
out into a typical module.
You’ll typically see shared definitions in a module known as
varieties.ts
, though I choose a extra contextual identify like
area.ts
which provides some hints concerning the type of varieties
contained therein.
On this case, I’m not assured that these varieties are actually the
similar. They is perhaps totally different projections of the identical area entity with
totally different fields, and I do not wish to share them throughout the
module boundaries risking deeper coupling. As unintuitive as this will likely
appear, I consider it’s the proper alternative: collapsing the entities is
very low cost and simple at this level. If they start to diverge, I in all probability
should not merge them anyway, however pulling them aside as soon as they’re sure
could be very tough.
If it appears to be like like a duck
I promised to clarify why I typically select to not export varieties.
I wish to make a sort out there to a different module provided that
I’m assured that doing so will not create incidental coupling, proscribing
the power of the code to evolve. Fortunately, Typescript’s structural or “duck” typing makes it very
straightforward to maintain modules decoupled whereas on the similar time guaranteeing that
contracts are intact at compile time, even when the kinds usually are not shared.
So long as the kinds are suitable in each the caller and callee, the
code will compile.
A extra inflexible language like Java or C# forces you into making some
selections earlier within the course of. For instance, when implementing
the scores algorithm, I’d be compelled to take a distinct method:
- I may extract the
RestaurantRating
kind to make it
out there to each the module containing the algorithm and the one
containing the general top-rated workflow. The draw back is that different
capabilities may bind to it, rising module coupling. - Alternatively, I may create two totally different
RestaurantRating
varieties, then present an adapter operate
for translating between these two similar varieties. This may be okay,
however it could enhance the quantity of template code simply to inform
the compiler what you want it already knew. - I may collapse the algorithm into the
topRated
module utterly, however that may give it extra
obligations than I would really like.
The rigidity of the language can imply extra pricey tradeoffs with an
method like this. In his 2004 article on dependency
injection and repair locator patterns, Martin Fowler talks about utilizing a
function interface to cut back coupling
of dependencies in Java regardless of the shortage of structural varieties or first
order capabilities. I’d positively think about this method if I had been
working in Java.
In abstract
By selecting to satisfy dependency contracts with capabilities fairly than
lessons, minimizing the code sharing between modules and driving the
design by exams, I can create a system composed of extremely discrete,
evolvable, however nonetheless type-safe modules. If in case you have related priorities in
your subsequent challenge, think about adopting some facets of the method I’ve
outlined. Remember, nevertheless, that selecting a foundational method for
your challenge isn’t so simple as choosing the “finest apply” requires
taking into consideration different elements, such because the idioms of your tech stack and the
abilities of your crew. There are various methods to
put a system collectively, every with a fancy set of tradeoffs. That makes software program structure
typically troublesome and all the time partaking. I would not have it some other approach.
[ad_2]