[ad_1]
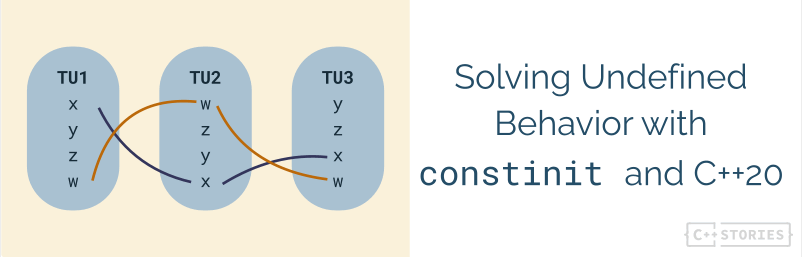
A number of years in the past (see right here, I confirmed an fascinating implementation for self-registering lessons in factories. It really works, however one step could be on the fringe of Undefined conduct. Fortuitously, with C++20, its new constinit
key phrase, we will replace the code and guarantee it’s tremendous protected.
Intro
Let’s convey again the subject:
Right here’s a typical manufacturing facility operate. It creates unique_ptr
with ZipCompression
or BZCompression
based mostly on the handed title/filename:
static unique_ptr<ICompressionMethod> Create(const string& fileName) {
auto extension = GetExtension(filename);
if (extension == "zip")
return make_unique<ZipCompression>();
else if (extension = "bz")
return make_unique<BZCompression>();
return nullptr;
}
Listed here are some points with this strategy:
- Every time you write a brand new class, and also you need to embody it within the manufacturing facility, it’s important to add one other if within the
Create()
technique. Simple to neglect in a posh system. - All the categories have to be recognized to the manufacturing facility.
- In
Create()
, we arbitrarily used strings to signify sorts. Such illustration is simply seen in that single technique. What if you happen to’d like to make use of it elsewhere? Strings could be simply misspelled, particularly if in case you have a number of locations the place they’re in contrast.
All in all, we get a powerful dependency between the manufacturing facility and the lessons.
However what if lessons may register themselves? Would that assist?
- The manufacturing facility would do its job: create new objects based mostly on some matching.
- If you happen to write a brand new class, there’s no want to alter components of the manufacturing facility class. Such a category would register mechanically.
Google Take a look at
To offer you extra motivation, I’d like to indicate one real-life instance. Whenever you use Google Take a look at library, and also you write:
TEST(MyModule, InitTest) {
// impl...
}
Behind this single TEST
macro, numerous issues occur! For starters, your take a look at is expanded right into a separate class – so every take a look at is a brand new class. However then, there’s an issue: you’ve gotten all of the checks, so how the take a look at runner is aware of about them? It’s the identical drawback had been’ making an attempt to unravel on this part. The lessons must be auto-registered.
Take a look at this code: from googletest/…/gtest-internal.h:
// (some components of the code minimize out)
#outline GTEST_TEST_(test_case_name, test_name, parent_class, parent_id)
class GTEST_TEST_CLASS_NAME_(test_case_name, test_name)
: public parent_class {
digital void TestBody();
static ::testing::TestInfo* const test_info_ GTEST_ATTRIBUTE_UNUSED_;
};
::testing::TestInfo* const GTEST_TEST_CLASS_NAME_(test_case_name, test_name)
::test_info_ =
::testing::inner::MakeAndRegisterTestInfo(
#test_case_name, #test_name, NULL, NULL,
new ::testing::inner::TestFactoryImpl<
GTEST_TEST_CLASS_NAME_(test_case_name, test_name)>);
void GTEST_TEST_CLASS_NAME_(test_case_name, test_name)::TestBody()
I minimize some components of the code to make it shorter, however principally, GTEST_TEST_
is used within the TEST
macro, and it will broaden to a brand new class. Within the decrease part, you may see the title MakeAndRegisterTestInfo
. So right here’s the place the place the category registers!
After the registration, the runner is aware of all the prevailing checks and might invoke them.
Implementing the manufacturing facility
Listed here are the steps to implement an analogous system:
- Some Interface – we’d prefer to create lessons derived from one interface. It’s the precise requirement as a “regular” manufacturing facility technique.
- Manufacturing unit class that additionally holds a map of accessible sorts.
- A proxy that shall be used to create a given class. The manufacturing facility doesn’t know easy methods to create a given kind now, so now we have to offer some proxy lessons.
For the interface, we will use ICompressionMethod
:
class ICompressionMethod {
public:
ICompressionMethod() = default;
digital ~ICompressionMethod() = default;
digital void Compress() = 0;
};
After which the manufacturing facility:
class CompressionMethodFactory {
public:
utilizing TCreateMethod = unique_ptr<ICompressionMethod>(*)();
public:
CompressionMethodFactory() = delete;
static bool Register(const string& title, TCreateMethod funcCreate);
static unique_ptr<ICompressionMethod> Create(const string& title);
personal:
static Map<string, TCreateMethod> s_methods;
};
The manufacturing facility holds the map of registered sorts. The principle level is that the manufacturing facility now makes use of some technique (TCreateMethod
) to create the specified kind (our proxy). The title of a sort and that creation technique have to be initialized in a special place.
The implementation of the manufacturing facility:
class CompressionMethodFactory {
public:
utilizing TCreateMethod = unique_ptr<ICompressionMethod>(*)();
public:
CompressionMethodFactory() = delete;
static constexpr bool Register(string_view title,
TCreateMethod createFunc) {
if (auto val = s_methods.at(title, nullptr); val == nullptr) {
if (s_methods.insert(title, createFunc)) {
std::cout << title << " registeredn";
return true;
}
}
return false;
}
static std::unique_ptr<ICompressionMethod> Create(string_view title) {
if (auto val = s_methods.at(title, nullptr); val != nullptr) {
std::cout << "calling " << title << "n";
return val();
}
return nullptr;
}
personal:
static inline constinit Map<string_view, TCreateMethod, 4> s_methods;
};
Now we will implement a derived class from ICompressionMethod
that may register within the manufacturing facility:
class ZipCompression : public ICompressionMethod {
public:
digital void Compress() override;
static unique_ptr<ICompressionMethod> CreateMethod() {
return std::make_unique<ZipCompression>();
}
static string_view GetFactoryName() { return "ZIP"; }
personal:
static inline bool s_registered =
CompressionMethodFactory::Register(ZipCompression::GetFactoryName(),
CreateMethod);
};
The draw back of self-registration is that there’s a bit extra work for a category. As you’ll be able to see, we will need to have a static CreateMethod
outlined.
To register such a category, all now we have to do is to outline s_registered
:
bool ZipCompression::s_registered =
CompressionMethodFactory::Register(ZipCompression::GetFactoryName(),
ZipCompression::CreateMethod);
The fundamental thought for this mechanism is that we depend on static variables. They are going to be initialized earlier than important()
is known as by way of dynamic initialization.
As a result of the order of initialization of static variables in several compilation models is unspecified, we’d find yourself with a special order of components within the manufacturing facility container. Every title/kind just isn’t depending on different already registered sorts in our instance, so we’re protected right here.
Tough case with the map
However what concerning the first insertion? Can we make sure that the Map
is created and prepared to be used?
I requested this query at SO: C++ static initialization order: including right into a map – Stack Overflow. Right here’s the tough abstract:
The conduct on this situation is technically undefined, in accordance with the C++ commonplace, as a result of the initialization order of static variables throughout completely different translation models (i.e., .cpp recordsdata) just isn’t specified. The map
Manufacturing unit::s_map
and the static variable registered are in several translation models within the instance. In consequence, it’s doable for the registered variable to be initialized (and thusManufacturing unit::Register
to be known as) earlier thanManufacturing unit::s_map
is initialized. If this occurs, this system will try and insert a component into an uninitialized map, resulting in undefined conduct.
However in C++20, we will do higher.
What if we may drive the compiler to make use of fixed initialization for the map? That means, regardless of the order of compilation models, the worth shall be already current and able to use.
This may be achieved by way of the constinit
key phrase. I described it in my different put up, however for a fast abstract:
This new key phrase for C++20 forces fixed initialization. It’ll make sure that the worth will already be current and initialized regardless of the compilation order. What’s extra, versus
constexpr
, we solely drive initialization, and the variable itself just isn’t fixed. So you’ll be able to change it later.
I applied a particular model of Map
, which has a constexpr
constructor (implicit) and, because of constinit
, shall be initialized earlier than s_registered
is initialized (for some first registered lessons).
My present implementation makes use of std::array
, which can be utilized in fixed expressions. We may probably use std::map,
however it might be on the fringe of Undefined Habits, so it’s not assured to work. Within the last code, it’s also possible to experiment with std::vector
, which received constexpr
help in C++20.
template <typename Key, typename Worth, size_t Dimension>
struct Map {
std::array<std::pair<Key, Worth>, Dimension> knowledge;
size_t slot_ { 0 };
constexpr bool insert(const Key &key, const Worth& val) {
if (slot_ < Dimension) {
knowledge[slot_] = std::make_pair(key, val);
++slot_;
return true;
}
return false;
}
[[nodiscard]] constexpr Worth at(const Key &key, const Worth& none) const {
const auto itr =
std::find_if(start(knowledge), finish(knowledge),
[&key](const auto &v) { return v.first == key; });
if (itr != finish(knowledge)) {
return itr->second;
} else {
return none;
}
}
};
And the Manufacturing unit
class CompressionMethodFactory {
public:
utilizing TCreateMethod = std::unique_ptr<ICompressionMethod>(*)();
public:
CompressionMethodFactory() = delete;
static constexpr bool Register(std::string_view title, TCreateMethod createFunc) {
if (auto val = s_methods.at(title, nullptr); val == nullptr) {
if (s_methods.insert(title, createFunc)) {
std::cout << title << " registeredn";
return true;
}
}
return false;
}
static std::unique_ptr<ICompressionMethod> Create(std::string_view title) {
if (auto val = s_methods.at(title, nullptr); val != nullptr) {
std::cout << "calling " << title << "n";
return val();
}
return nullptr;
}
personal:
static inline constinit Map<std::string_view, TCreateMethod, 4> s_methods;
};
Optimizing s_registered
?
We also needs to ask one query: Can the compiler remove s_registered
? Fortuitously, we’re additionally on the protected aspect. From the most recent draft of C++: [basic.stc.static#2]:
If a variable with static storage period has initialization or a destructor with unwanted side effects, it shall not be eradicated even when it seems to be unused, besides {that a} class object or its copy/transfer could also be eradicated as laid out in class.copy.elision.
Since s_registered
has an initialization with unwanted side effects (calling Register()
), the compiler can’t optimize it.
(See the way it works in a library: Static Variables Initialization in a Static Library, Instance – C++ Tales)
Closing Demo
See the complete instance @Wandbox
#embody "ICompressionMethod.h"
#embody "ZipCompression.h"
#embody <iostream>
int important() {
std::cout << "important begins...n";
if (auto pMethod = CompressionMethodFactory::Create("ZIP"); pMethod)
pMethod->Compress();
else
std::cout << "Can't discover ZIP...n";
if (auto pMethod = CompressionMethodFactory::Create("BZ"); pMethod)
pMethod->Compress();
else
std::cout << "Can't discover BZ...n";
if (auto pMethod = CompressionMethodFactory::Create("7Z"); pMethod)
pMethod->Compress();
else
std::cout << "Can't discover 7Z...n";
}
Right here’s the sequence diagram for this demo:
The anticipated output:
evaluating: ZIP to
evaluating: ZIP to
evaluating: ZIP to
evaluating: ZIP to
inserting at 0
ZIP|0x5586bceb06f0
ZIP registered
evaluating: BZ to ZIP
evaluating: BZ to
evaluating: BZ to
evaluating: BZ to
inserting at 1
BZ|0x5586bceb21f0
BZ registered
important begins...
evaluating: ZIP to ZIP
calling ZIP
Zip compression...
evaluating: BZ to ZIP
evaluating: BZ to BZ
calling BZ
BZ compression...
evaluating: 7Z to ZIP
evaluating: 7Z to BZ
evaluating: 7Z to
evaluating: 7Z to
Can't discover 7Z...
Extra within the guide
Study extra about numerous initialization guidelines, tips and examples in my guide:
Print model @Amazon
C++ Initialization Story E-book @Leanpub
Abstract
On this weblog put up, we delved into the intricate particulars of a way for creating self-registering lessons in C++20, enabling the creation of extra versatile and scalable manufacturing facility patterns. A central element of this answer is the constinit
key phrase, a function launched in C++20 that ensures the initialization of a variable at compile-time.
Our strategy begins with the standard manufacturing facility operate, which was proven to have some inherent shortcomings, particularly: the necessity to replace the manufacturing facility with every new class, the manufacturing facility’s requirement to concentrate on every kind, and the dependency between the manufacturing facility and the lessons.
To beat these points, we constructed a self-registration system impressed by how Google Take a look at handles its take a look at circumstances. The system consists of an interface from which the lessons are derived, a manufacturing facility class that holds a map of accessible sorts, and a proxy used to create a given class. The mechanism depends on static variables, that are initialized earlier than the important()
operate is known as.
Nevertheless, the order of initialization of static variables throughout completely different compilation models is unspecified, which may result in points. The central trick to avoiding these issues lies within the constinit
key phrase, making certain that the Map
we use to carry the registered lessons is initialized at compile-time, earlier than any static class registration happens.
We demonstrated how this mechanism works by way of the instance of a CompressionMethodFactory
and the ZipCompression
class. The system works in a means that every class registers itself within the manufacturing facility, thus negating the necessity for the manufacturing facility to have prior information about it.
Through the use of constinit
, we make sure that our system will behave constantly, whatever the order through which static variables are initialized. This function, coupled with a easy but efficient map implementation that makes use of a constexpr
constructor, ensures our strategy is protected and effectively throughout the realm of outlined conduct.
In conclusion, the usage of constinit
in C++20 gives a strong means to handle static initialization order, creating alternatives for extra sturdy and maintainable code constructions. It supplies a powerful basis for self-registering lessons and factories, simplifying the method and decreasing dependency points. The approach explored on this put up signifies the energy of recent C++ options and their functionality to unravel complicated issues in a chic and environment friendly method.
Again to you
- Have you ever tried self-registering lessons?
- Have you learnt another patterns useful with factories?
Share your suggestions under within the feedback.
[ad_2]