[ad_1]
As a Python developer, you might need encountered the phrases async
and await
, questioning what they’re and the right way to use them in your initiatives.
Async capabilities allow you to put in writing concurrent code utilizing the async
/await
syntax. This highly effective duo means that you can carry out a number of duties concurrently with out blocking the execution of your code. ?️ Consider it as having a number of browser tabs open; whereas one web page hundreds, you may proceed shopping different tabs. This functionality means your Python purposes can turn out to be quicker, extra environment friendly, and able to dealing with many I/O operations. ?
To get began with async
capabilities, you’ll must get acquainted with Python’s asyncio
library, which serves as a basis for quite a few asynchronous frameworks resembling high-performance community and internet servers, database connection libraries, and distributed activity queues. ?
Mastering async capabilities can actually elevate your Python programming abilities and aid you construct highly effective and responsive purposes. ?
Python Async Perform Fundamentals

First, you’ll study the fundamentals of Python async
capabilities, which can assist enhance the efficiency of your asynchronous programming.
We’ll cowl
- async capabilities with out
await
, - an async perform instance, async perform return, and
- async perform name.
Async Perform With out Await
You would possibly marvel if it’s potential to create an async perform with out utilizing the await
key phrase. Effectively, it’s!
Nevertheless, with out await
, the async perform turns into considerably much less helpful, because you gained’t have the ability to pause its execution and yield management again to the occasion loop.
This implies your async code won’t be able to realize cooperative concurrency, and different coroutines is perhaps caught ready for his or her flip to execute. It’s usually a good suggestion to make use of await
when working with async capabilities for extra environment friendly asynchronous programming.
Async Perform Instance
Let’s dive right into a easy instance of utilizing an async perform in Python with asyncio
:
import asyncio async def greet(identify: str): print(f"Howdy, {identify}!") await asyncio.sleep(1) print(f"Good to fulfill you, {identify}!") async def predominant(): task1 = asyncio.create_task(greet("Alice")) task2 = asyncio.create_task(greet("Bob")) await task1 await task2 asyncio.run(predominant())

On this instance, an async perform greet
is asserted, which prints a greeting message, waits for 1 second utilizing asyncio.sleep
, after which prints one other message.
The predominant
asynchronous perform creates two duties to name greet
with totally different names, operating them concurrently.
Async Perform Return
While you wish to return a worth from an async perform, simply use the return
assertion as you’ll in common capabilities. Nevertheless, remember the fact that the returned worth will likely be wrapped in an asyncio.Future
object, not the precise worth.
You’ll want to make use of await
to get the worth when calling this async perform.
For instance:
async def calculate_result(): await asyncio.sleep(1) return "End result!" async def predominant(): consequence = await calculate_result() print(consequence) asyncio.run(predominant())
Right here, calculate_result
is an async perform that returns a worth after asynchronously ready for 1 second. Within the predominant()
perform, you should use await
to get the precise worth and print it. ?
Async Perform Name
To name an async perform, you may’t merely use the conventional perform name syntax, as a result of doing so would simply return a coroutine object, not the precise results of the perform. As an alternative, it’s important to use the await
key phrase to name the async perform, or use asyncio.create_task
or related capabilities to run it concurrently:
# Utilizing `await` to name async perform consequence = await async_function() # Utilizing `asyncio.create_task` to run concurrently activity = asyncio.create_task(async_function())
Keep in mind to at all times use the suitable methodology to name your async capabilities with the intention to obtain environment friendly asynchronous programming with Python’s highly effective async/await
syntax.
Superior Async Perform Ideas

Subsequent, you’ll discover superior async perform ideas to present you a greater understanding of how they work in Python. ? Prepared? Let’s dive in!
Async Perform Decorator
To create an async perform, you’ll use the async def
syntax. This implies you don’t have to make use of a decorator, however you may nonetheless enhance asynchronous capabilities with the @some_decorator
syntax for higher modularity in your packages.
As an example, think about using @asyncio.coroutine
with a yield from
syntax for those who’re working with Python 3.4 or earlier. Or just improve to newer variations! ?
Async Perform Sort Trace
Sort hints assist enhance the readability of your async code. Specify the enter and output sorts of your async perform utilizing the typing
module’s Coroutine and asyncio
‘s Future objects.
Right here’s an instance:
from typing import Coroutine import asyncio async def some_async_function() -> Coroutine[str]: await asyncio.sleep(1) return "achieved"
Async Perform Returns Coroutine
An async perform, often known as a coroutine, returns a coroutine object when known as. You need to use it as a direct name or cross it to an occasion loop to run the async perform utilizing asyncio.run()
or loop.run_until_complete()
.
Consider coroutine objects aren’t executed till you explicitly use an occasion loop or an await
expression.
Async Perform Await
When writing async capabilities, the await
key phrase is essential. It means that you can pause the execution of a coroutine and look ahead to a consequence with out blocking different coroutines.
You’ll typically use await
with I/O-bound operations, like studying from recordsdata, interacting with community providers, or retrieving sources, which may take a big period of time.
⭐ Advisable: Python __await()__
Magic Technique
Async Perform Sort
An async perform’s kind is coroutine
. So when defining your async perform, you’re primarily making a non-blocking perform that enables different capabilities to run whereas it waits for outcomes.
To examine if an object in Python is a coroutine, you should use examine.iscoroutine(obj)
or examine.iscoroutinefunction(obj)
.
Async Perform Return Sort
Async capabilities return a coroutine
object, however you too can specify the kind of the eventual returned worth. As an example, in case your async perform performs some networking duties and returns JSON knowledge, you may specify the return kind as Dict[str, Any]
.
Right here’s the way you try this:
from typing import Dict, Any, Coroutine import asyncio async def fetch_json_data() -> Coroutine[Dict[str, Any]]: # some networking duties right here await asyncio.sleep(2) return {"key": "worth"}
Async Perform in Completely different Contexts

On this part, we are going to discover utilizing async capabilities in numerous circumstances, resembling inside courses, threads, and changing async capabilities to sync. We will even talk about the ideas of async perform sleep and dealing with capabilities that have been by no means awaited.
Async Perform in Class
When working with courses in Python, you would possibly wish to embrace asynchronous strategies. To attain this, simply outline your class methodology with async def
. Keep in mind to await
your async strategies when calling them to make sure correct execution.
Right here’s an instance:
class MyClass: async def my_async_method(self): await asyncio.sleep(1) async def predominant(): my_obj = MyClass() await my_obj.my_async_method()
Async Perform in Thread
Operating async capabilities in a thread may be difficult as a consequence of occasion loop necessities. Use asyncio.to_thread()
for operating async capabilities in threads. This may guarantee your async perform is executed throughout the right thread’s occasion loop.
For instance:
async def my_async_function(): await asyncio.sleep(1) print("Howdy from async perform!") async def predominant(): consequence = await asyncio.to_thread(my_async_function)
Async Perform to Sync
If you’ll want to name an async perform from synchronous code, you should use asyncio.run()
or create an occasion loop that runs a given coroutine.
Right here’s an instance of the right way to run an async perform from sync code:
def sync_function(): asyncio.run(my_async_function())
Async Perform Sleep
Typically, you would possibly wish to introduce a delay in your coroutine utilizing asyncio.sleep
. This permits different coroutines to run whereas ready for IO operations or different occasions.
Instance:
async def delayed_hello(): await asyncio.sleep(1) print("Howdy after 1 second!")
? Advisable: Time Delay in Python
Async Perform Was By no means Awaited
In some instances, it’s possible you’ll neglect to await
an async perform, which results in warnings resembling "coroutine 'my_async_function' was by no means awaited."
To stop these points, at all times make sure you’re utilizing await
when calling async capabilities:
async def my_async_function(): await asyncio.sleep(1) async def predominant(): # Lacking 'await' would result in a warning await my_async_function()
When you acquired one thing out of this text, I’m positive you’ll study one thing of this one: ?
? Advisable: Python Async With Assertion — Simplifying Asynchronous Code
I promise it has extra stunning pics. ?
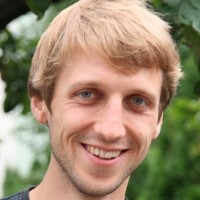
Whereas working as a researcher in distributed methods, Dr. Christian Mayer discovered his love for educating pc science college students.
To assist college students attain greater ranges of Python success, he based the programming schooling web site Finxter.com that has taught exponential abilities to hundreds of thousands of coders worldwide. He’s the creator of the best-selling programming books Python One-Liners (NoStarch 2020), The Artwork of Clear Code (NoStarch 2022), and The Guide of Sprint (NoStarch 2022). Chris additionally coauthored the Espresso Break Python collection of self-published books. He’s a pc science fanatic, freelancer, and proprietor of one of many prime 10 largest Python blogs worldwide.
His passions are writing, studying, and coding. However his best ardour is to serve aspiring coders by means of Finxter and assist them to spice up their abilities. You’ll be able to be part of his free e-mail academy right here.
[ad_2]