[ad_1]
Python Lookahead
You would possibly end up working with common expressions in Python, and the lookahead characteristic is sort of helpful in lots of conditions.
Lookahead is available in two flavors: optimistic lookahead and adverse lookahead. Let’s discover these kind of lookahead and see how you should utilize them in your Python code! ?
Optimistic lookahead is an assertion that checks whether or not a given sample is adopted by one other sample. In Python, that is finished utilizing the (?=)
syntax. If it’s profitable, the regex engine continues matching, but when not, it continues looking from the place it left off.
Contemplate this instance:
import re textual content = "I like coding in Python!" sample = r"bw+(?=ing)" end result = re.findall(sample, textual content) print(end result) # Output: ['cod']
Right here, we seek for a phrase ending in "ing"
however solely return the phrase with out the "ing"
half. The optimistic lookahead makes certain that the phrase is adopted by "ing"
, but it surely doesn’t embody these characters within the precise match. ?
Now let’s take a look at adverse lookahead, which asserts {that a} given sample is not adopted by one other sample. You utilize the (?!sample)
syntax in Python for this objective.
Check out this instance to see the way it works:
sample = r"bw+b(?!ing)" end result = re.findall(sample, textual content) print(end result) # Output: ['I', 'love', 'in', 'Python!']
On this case, we discover all phrases not adopted by "ing"
. Discover that the "cod"
a part of "coding"
is lacking, because the adverse lookahead assertion checks for the absence of "ing"
after the phrase.?
You too can use a number of lookaheads, each optimistic or adverse, to create extra complicated patterns:
sample = r"bw+(?=ing)(?!Python)" end result = re.findall(sample, textual content) print(end result) # Output: []
Right here, we use a optimistic lookahead to seek out phrases that finish in "ing"
and a adverse lookahead to exclude matches with "Python"
. On this instance, nothing matches the standards, so the result’s an empty checklist. ?
? Do not forget that lookahead assertions don’t devour any characters within the matching course of, making them zero-width assertions. This lets you carry out superior searches in your strings with out affecting the matched content material.
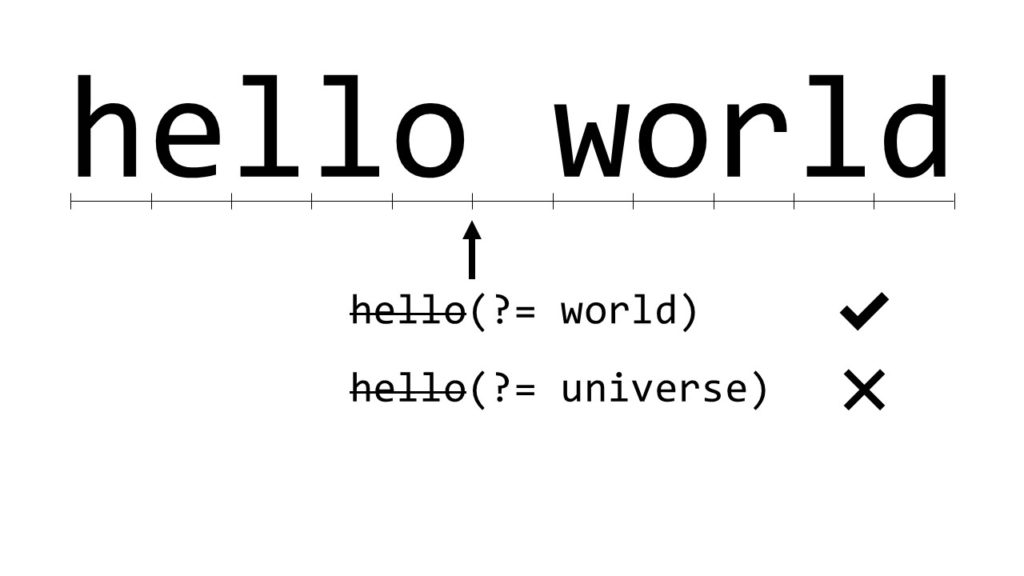
Determine: A easy instance of lookahead. The common expression engine matches (“consumes”) the string partially. Then it checks whether or not the remaining sample could possibly be matched with out truly matching it.
Lookahead assertions in Python regexes assist you match string patterns which can be both adopted by (optimistic lookahead) or not adopted by (adverse lookahead) a specified sample. ?
Python Lookahead Instance ?

Let’s dive into the optimistic lookahead syntax: (?=Y)
. This syntax means you need to seek for a sample however matches solely whether it is adopted by one other sample.
Contemplate the next code snippet that demonstrates this idea in motion:
import re string = "I like Python3 and Python2" sample = r'Python(?=d)' matches = re.findall(sample, string) print(matches) # Output: ['Python', 'Python']
Within the instance above, you’re looking for the phrase "Python"
solely whether it is adopted by a digit. The optimistic lookahead doesn’t embody the digit within the match, so the output is an inventory containing simply the phrase "Python"
twice. ?
However, adverse lookahead makes use of the syntax (?!Y)
and matches a sample solely whether it is NOT adopted by one other sample.
Let’s check out an instance:
sample = r'Python(?!3)' matches = re.findall(sample, string) print(matches) # Output: ['Python']
On this instance, you’re looking for the phrase "Python"
not adopted by the digit '3'
. The output comprises only one match, which is the "Python"
adopted by the digit '2'
. ?
A number of Lookahead – Optimistic and Damaging

At this level, you already know that lookaheads in regex are a robust method to make sure a sure sample is instantly adopted by one other sample. Optimistic lookaheads be sure the sample is there, whereas adverse lookaheads make sure the sample is not there.
Let’s dive into utilizing a number of lookaheads in Python.
A number of Optimistic Lookaheads
Suppose you need to discover some textual content in a string that’s adopted by a number of particular patterns. You may mix a number of optimistic lookaheads like this:
X(?=Y)(?=Z)
For instance, let’s say you’re looking for a phrase adopted by a digit after which by a capital letter.
Your regex may appear to be this:
import re sample = r'bw+(?=d)(?=[A-Z])' string = "Python3A Tutorial4B" matches = re.findall(sample, string)
Right here, the regex bw+(?=d)(?=[A-Z])
searches for phrases adopted by a digit after which by a capital letter. On this case, there are not any matches as a result of no phrases meet these circumstances.
A number of Damaging Lookaheads
Equally, you should utilize a number of adverse lookaheads to make sure that your sample isn’t adopted by sure patterns. The syntax is just like optimistic lookaheads:
X(?!Y)(?!Z)
For example, let’s discover a phrase that’s not adopted by a digit and likewise not adopted by a capital letter:
import re pattern2 = r'bw+(?!d)(?![A-Z])' string = "Python3A Tutorial4B" matches2 = re.findall(pattern2, string)
On this case, the regex bw+(?!d)(?![A-Z])
searches for phrases not adopted by a digit and never adopted by a capital letter. The end result might be ['Python', 'Tutorial']
.
By the way in which, it’s possible you’ll have an interest on this tutorial on regex teams and lookahead:
Widespread Points with Lookahead

Python Optimistic Lookahead Not Working
? So that you’re making an attempt to make use of a optimistic lookahead in your Python regex, but it surely’s not working as anticipated? Don’t fear! More often than not, this may be resolved by double-checking your regex sample.
Be sure that the syntax is right: (?=lookahead_regex)
(supply).
Additionally, remember that lookahead assertions are zero-width, which means they don’t devour enter characters. So double-check your use of group assertions and quantifiers!
# Correct utilization of optimistic lookahead sample = r"abc(?=d)"
? Bear in mind, optimistic lookahead checks if the string instantly following the present place matches a particular sample.
Python Damaging Lookahead Not Working
? Having bother with adverse lookahead in Python regex?
As soon as once more, the secret’s guaranteeing that your regex sample follows the right syntax: (?!lookahead_regex)
(supply).
Damaging lookahead means the regex engine will exclude matching patterns in the event that they instantly observe the present place.
# Proper method to make use of adverse lookahead sample = r"abc(?!def)"
? Be aware that adverse lookahead assertions are additionally zero-width. Widespread errors can embody improper use of group assertions or quantifiers at the side of lookahead.
Splitting patterns into a number of lookaheads or lookahead+lookbehind pairs will help remedy complicated matching necessities extra successfully.
Utilizing Lookahead in Varied Features
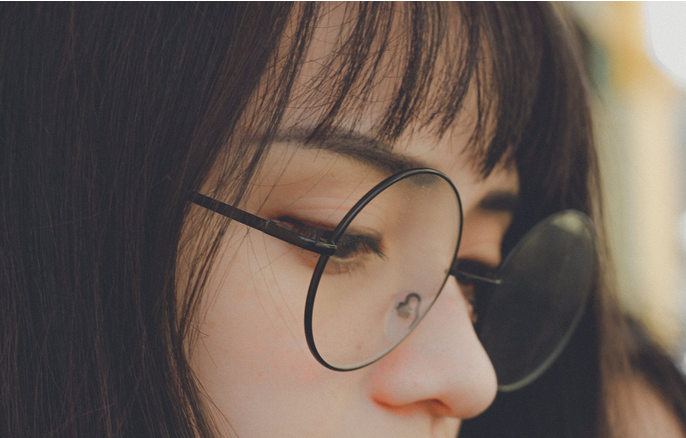
Let’s talk about find out how to use lookahead in numerous Python regex features, similar to re.findall()
, re.sub()
, re.match()
, and re.change()
.
Python re.findall() Lookahead ?
When it is advisable to discover all occurrences of a sample with lookahead, the re.findall()
perform is your finest pal.
import re sample = r'meals(?=ie)' textual content = "foodie and junkfoodie and seafoodie" end result = re.findall(sample, textual content) print(end result) # Output: ['food', 'food']
On this instance, you’re looking for the phrase 'meals'
adopted by 'ie'
with out together with 'ie'
within the end result. The re.findall()
perform makes it simple to extract this info.
? Really useful: Python Regex Findall Perform
Python re.sub() Lookahead ?
To carry out a search-and-replace operation whereas contemplating lookahead, you should utilize the re.sub()
perform.
sample = r'meals(?=ie)' textual content = "foodie and junkfoodie and seafoodie" end result = re.sub(sample, '***', textual content) print(end result) # Output: "***ie and junk***ie and sea***ie"
Right here, you’re changing the phrase 'meals'
with asterisks (***
) solely when it’s adopted by 'ie'
, with out together with 'ie'
within the alternative.
? Really useful: Python Regex Sub Perform
Python re.match() Lookahead ?
re.match()
can be utilized with lookahead to examine if the enter string begins with the required sample.
sample = r'(?=foodie)' textual content = "foodie and junkfoodie and seafoodie" end result = re.match(sample, textual content) print(bool(end result)) # Output: True
This instance checks whether or not the string begins with 'foodie'
with out together with it within the end result.
? Really useful: Python Regex Match Perform
Python Lookaround
Lookarounds in a regex are highly effective zero-width assertions that help you peek round your search string to discover a match with out together with surrounding characters. They arrive in two fundamental flavors: lookahead and lookbehind ?.

Lookahead
? Optimistic lookahead syntax: (?=<lookahead_regex>)
? Damaging lookahead syntax: (?!<lookahead_regex>)
Lookaheads examine in case your sample happens instantly forward (to the best) of the parser’s present place. For instance, let’s discover all alphanumeric characters adopted by a decimal digit ?.
import re sample = r'w(?=d)' textual content="A1B2C3D4" matches = [match.group() for match in re.finditer(pattern, text)] print(matches) # ['A', 'B', 'C']
Utilizing (?=d)
as a optimistic lookahead, we solely matched alphanumeric characters immediately previous a decimal digit ?.
Lookbehind
? Optimistic lookbehind syntax: (?<=<lookbehind_regex>)
? Damaging lookbehind syntax: (?<!<lookbehind_regex>)
Optimistic lookbehinds, then again, examine the situation to the left of the present place. For instance, let’s discover all whitespace characters after a literal dot ?️♀️.
sample = r'(?<=.)s' textual content="Hiya. World! How.are.you?" matches = [match.group() for match in re.finditer(pattern, text)] print(matches) # [' ']
Using (?<=.)
as a optimistic lookbehind, we efficiently matched solely the whitespace character following a literal dot ?.
Frequent Questions
On this part, we’ll talk about some frequent questions you would possibly encounter when working with Python regex lookahead. We’ll present options and examples for every drawback, so you’ll be able to deal with them extra confidently in your personal initiatives. ?
Python Lookahead vs Lookbehind — What’s the Distinction?
In a nutshell, lookahead checks if a sure sample follows your match, however doesn’t devour characters, whereas lookbehind checks if a sure sample comes earlier than your match, and doesn’t devour characters both.
? So, should you’re making an attempt to establish a sample that seems after your present match, use lookahead; should you’re searching for a sample earlier than your match, use lookbehind.
Python Regex Non-Grasping Lookahead
In order for you your lookahead to be non-greedy (match as few characters as doable), you should utilize the *?
quantifier.
? For instance, if you wish to match a string that’s adopted by any variety of areas, however not adopted by a comma, you may use the regex '.*?(?=s*)'
.
This may match any sequence of characters, however cease matching as quickly because it reaches an area or a comma.
Python Regex Lookbehind Variable Size
Python regex lookbehind does not assist variable-length patterns, so if it is advisable to use lookbehind with a variable-length sample, you’ll have to seek out one other strategy.
One widespread workaround is to make use of a non-capturing group adopted by a lookbehind, like '(?:<pattern1>|<pattern2>)(?<=<pattern2>)'
. This may help you match a variable-length sample utilizing lookbehind.
Python Regex Lookbehind Fastened Width
When you’re utilizing a fixed-width sample in your lookbehind, issues are a lot easier. You may merely use (?<=<sample>)
syntax to realize this.
? For instance, to match a three-digit quantity preceded by “No.”, you may use (?<=No.)d{3}
. You’ll solely match the three-digit quantity, however the "No."
half received’t be included within the match.
Python Regex Lookahead Finish of String
To examine in case your sample is adopted by the tip of the string, you should utilize the $
anchor in your lookahead.
? For instance, if you wish to match a sequence of digits provided that it’s on the finish of a string, you should utilize 'd+(?=$)'
. This may match a number of digits, however provided that they’re adopted by the tip of the string.
Python Regex Conditional Lookahead
Conditional lookahead permits you to specify completely different patterns primarily based on whether or not your lookahead succeeds or fails. To make use of conditional lookahead in Python regex, you should utilize the (?:(?=A)X|Y)
syntax. If the lookahead A
succeeds, the sample X
might be tried; if it fails, the sample Y
might be tried.
? For instance, (?:(?=d)Even|Odd)
will match the phrase "Even"
if it’s adopted by a digit, and "Odd"
in any other case.
Python Lookahead Iterator and Assertion (+Instance)
Utilizing a lookahead iterator means you’re looping over the matches utilizing a lookahead assertion.
For instance, if you wish to match all situations of the phrase "apple"
which can be adopted by an area however not embody the areas within the match, you should utilize the next strategy:
import re textual content = "I like apple , however not as a lot as apple pie." sample = re.compile(r"apple(?=s)") matches = [m.group(0) for m in pattern.finditer(text)] print(matches) # Output: ['apple', 'apple']
This instance makes use of a lookahead assertion (?=s)
to make sure "apple"
is adopted by an area, and an iterator to loop over all matches.
? Really useful: Python Regex Superpower (Full Information)
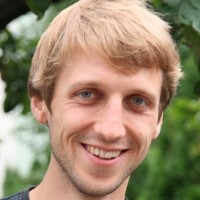
Whereas working as a researcher in distributed programs, Dr. Christian Mayer discovered his love for instructing pc science college students.
To assist college students attain increased ranges of Python success, he based the programming schooling web site Finxter.com that has taught exponential expertise to thousands and thousands of coders worldwide. He’s the writer of the best-selling programming books Python One-Liners (NoStarch 2020), The Artwork of Clear Code (NoStarch 2022), and The E-book of Sprint (NoStarch 2022). Chris additionally coauthored the Espresso Break Python collection of self-published books. He’s a pc science fanatic, freelancer, and proprietor of one of many prime 10 largest Python blogs worldwide.
His passions are writing, studying, and coding. However his biggest ardour is to serve aspiring coders by way of Finxter and assist them to spice up their expertise. You may be part of his free e mail academy right here.
[ad_2]